C#: Check whether two integers are equal or not
Write a C# Sharp program to accept two integers and check whether they are equal or not.
Sample Solution:-
C# Sharp Code:
using System; // Importing the System namespace
public class Exercise1 // Declaration of the Exercise1 class
{
public static void Main() // Entry point of the program
{
int int1, int2; // Declaration of integer variables int1 and int2
Console.Write("\n\n"); // Printing new lines
Console.Write("Check whether two integers are equal or not:\n"); // Displaying the purpose of the program
Console.Write("-------------------------------------------"); // Displaying a separator
Console.Write("\n\n"); // Printing new lines
Console.Write("Input 1st number: "); // Prompting user to input the first number
int1 = Convert.ToInt32(Console.ReadLine()); // Reading the first input number from the user
Console.Write("Input 2nd number: "); // Prompting user to input the second number
int2 = Convert.ToInt32(Console.ReadLine()); // Reading the second input number from the user
if (int1 == int2) // Checking if int1 is equal to int2
Console.WriteLine("{0} and {1} are equal.\n", int1, int2); // Printing a message if the numbers are equal
else
Console.WriteLine("{0} and {1} are not equal.\n", int1, int2); // Printing a message if the numbers are not equal
}
}
Sample Output:
Check whether two integers are equal or not: ------------------------------------------- Input 1st number: 20 Input 2nd number: 20 20 and 20 are equal.
Pictorial Presentation:
Flowchart :
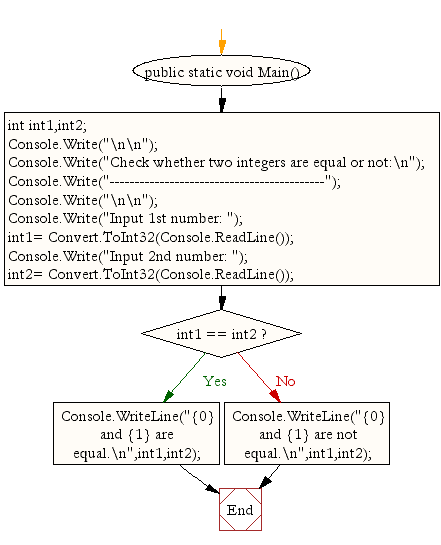
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: C# Sharp Conditional Statement Exercise.
Next: Write a C program to check whether a given number is even or odd.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.