C#: Check whether a number is even or odd
Write a C program to check whether a given number is even or odd.
Calculating a Even Numbers:
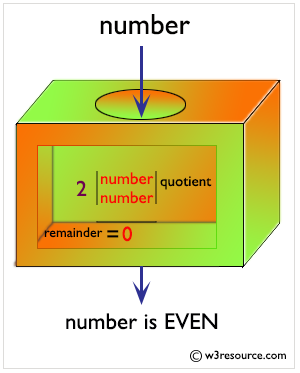
Even Numbers between 1 to 100:
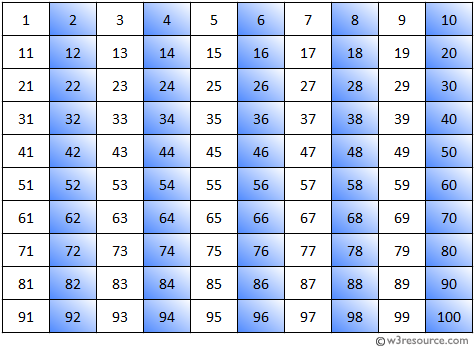
Calculating a Odd Numbers:
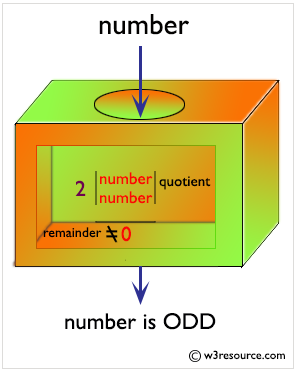
Odd Numbers between 1 to 100:
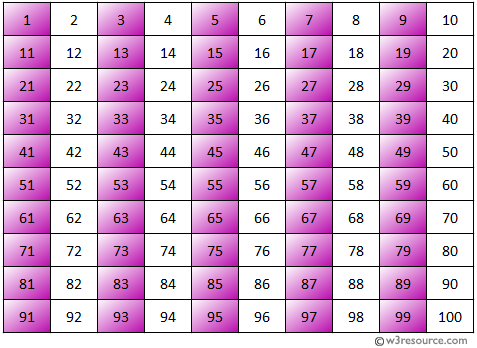
Sample Solution:-
C# Sharp Code:
using System; // Importing the System namespace
public class Exercise2 // Declaration of the Exercise2 class
{
public static void Main() // Entry point of the program
{
int num1, rem1; // Declaration of integer variables num1 and rem1
Console.Write("\n\n"); // Printing new lines
Console.Write("Check whether a number is even or odd :\n"); // Displaying the purpose of the program
Console.Write("---------------------------------------"); // Displaying a separator
Console.Write("\n\n"); // Printing new lines
Console.Write("Input an integer : "); // Prompting user to input an integer
num1 = Convert.ToInt32(Console.ReadLine()); // Reading the input integer from the user
rem1 = num1 % 2; // Calculating the remainder when num1 is divided by 2
if (rem1 == 0) // Checking if the remainder is equal to 0
Console.WriteLine("{0} is an even integer.\n", num1); // Printing a message if the number is even
else
Console.WriteLine("{0} is an odd integer.\n", num1); // Printing a message if the number is odd
}
}
Sample Output:
Check whether a number is even or odd : --------------------------------------- Input an integer : 10 10 is an even integer.
Flowchart:
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C# Sharp program to accept two integers and check whether they are equal or not.
Next: Write a C# Sharp program to check whether a given number is positive or negative.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.