C#: Perform an operation on +, -, *, x, / and displays the result of that operation
C# Sharp Basic Data Types: Exercise-4 with Solution
Write a C# Sharp program that takes two numbers as input and performs an operation (+,-,*,x,/) on them and displays the result of that operation.
Sample Solution:-
C# Sharp Code:
using System;
public class Exercise4
{
public static void Main()
{
// Declaration of variables for input
int x, y;
char operation;
// User input for the first number
Console.Write("Input first number: ");
x = Convert.ToInt32(Console.ReadLine());
// User input for the operation to perform (+, -, *, /)
Console.Write("Input operation: ");
operation = Convert.ToChar(Console.ReadLine());
// User input for the second number
Console.Write("Input second number: ");
y = Convert.ToInt32(Console.ReadLine());
// Conditionals to perform the arithmetic operation based on the operator entered
if (operation == '+')
Console.WriteLine("{0} + {1} = {2}", x, y, x + y);
else if (operation == '-')
Console.WriteLine("{0} - {1} = {2}", x, y, x - y);
else if ((operation == 'x') || (operation == '*'))
Console.WriteLine("{0} * {1} = {2}", x, y, x * y);
else if (operation == '/')
Console.WriteLine("{0} / {1} = {2}", x, y, x / y);
else
Console.WriteLine("Wrong Character"); // Displayed if an invalid operator is entered
}
}
Sample Output:
Input first number: 4 Input operation: * Input second number: 4 4 * 4 = 16
Visual Presentation:
Flowchart:
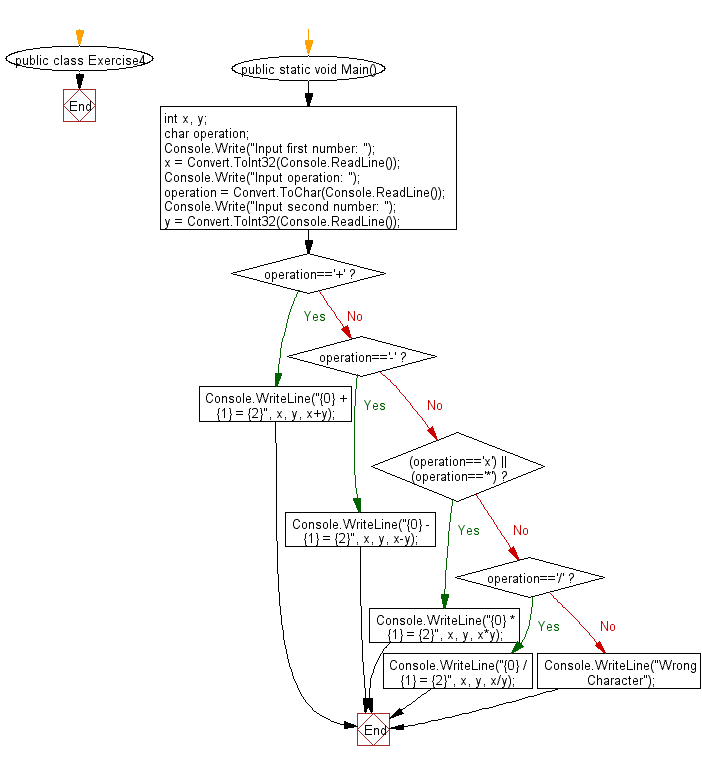
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C# Sharp program that takes userid and password as input (type string). After 3 wrong attempts, user will be rejected.
Next: Write a C# Sharp program that takes the radius of a circle as input and calculate the perimeter and area of the circle.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/csharp-exercises/data-types/csharp-data-type-exercise-4.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics