C#: Test a password
Write a C# Sharp program that takes userid and password as input (string type). After 3 unsuccessful attempts, the user will be rejected.
Sample Solution:-
C# Sharp Code:
using System;
public class Exercise3
{
public static void Main()
{
// Declaration of variables
string username, password;
int ctr = 0, dd = 0;
// Display instructions for username and password
Console.Write("\n\nCheck username and password :\n");
Console.Write("N.B. : Default username and password is: username and password\n");
Console.Write("---------------------------------\n");
// Loop for username and password input
do
{
// Prompt user to input a username
Console.Write("Input a username: ");
username = Console.ReadLine();
// Prompt user to input a password
Console.Write("Input a password: ");
password = Console.ReadLine();
// Check if the entered username and password match the default values
if (username == "username" && password == "password")
{
// Set dd flag to 1 if username and password match
dd = 1;
// Reset the attempt counter to 3 if the correct login is done
ctr = 3;
}
else
{
// Set dd flag to 0 if username and password don't match
dd = 0;
// Increment the attempt counter
ctr++;
}
}
while ((username != "username" || password != "password") && (ctr != 3));
// Display messages based on login attempt results
if (dd == 0)
{
// Display a message if login attempts exceed three times
Console.Write("\nLogin attempt more than three times. Try later!\n\n");
}
else if (dd == 1)
{
// Display a success message if password entered successfully
Console.Write("\nPassword entered successfully!\n\n");
}
}
}
Sample Output:
Input a username: robin Input a password: sds45 Input a username: john Input a password: dfgrt Input a username: doom Input a password: jkkl Login attemp more than three times. Try later!
Visual Presentation:
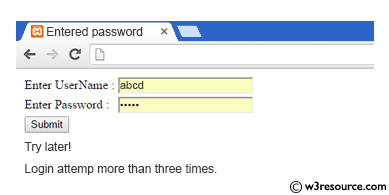
Flowchart:
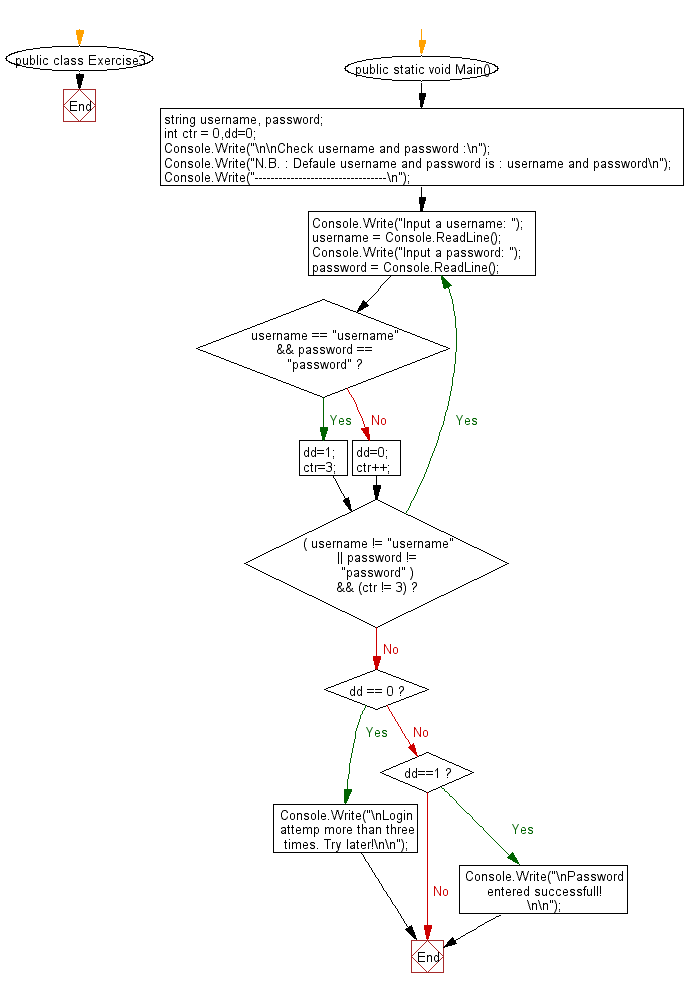
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C# Sharp program that takes a number and a width also a number, as input and then displays a triangle of that width, using that number.
Next: Write a C# Sharp program that takes two numbers as input and perform an operation (+,-,*,x,/) on them and displays the result of that operation.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.