C#: Calculate n terms of square natural number and their sum
C# Sharp For Loop: Exercise-25 with Solution
Write a C# Sharp program that displays the n terms of square natural numbers and their sum.
The series is as below:
Sample Solution:-
C# Sharp Code:
using System; // Importing necessary namespace
public class Exercise25 // Declaration of the Exercise25 class
{
public static void Main() // Main method, entry point of the program
{
int i, n, sum = 0; // Declaration of variables i, n, and sum as integers
Console.Write("\n\n"); // Displaying new lines
Console.Write("Calculate n terms of square natural number and their sum:\n"); // Displaying the purpose of the program
Console.Write("-----------------------------------------------------------"); // Displaying a separator
Console.Write("\n\n");
Console.Write("Input the number of terms : "); // Prompting the user to input the number of terms
n = Convert.ToInt32(Console.ReadLine()); // Reading the number of terms entered by the user
Console.Write("\nThe square natural upto {0} terms are :", n); // Displaying a message for square natural numbers
// Loop to calculate square natural numbers and their sum
for (i = 1; i <= n; i++)
{
Console.Write("{0} ", i * i); // Displaying the square of natural numbers
sum += i * i; // Calculating the sum of square natural numbers
}
// Displaying the sum of square natural numbers
Console.Write("\nThe Sum of Square Natural Number upto {0} terms = {1} \n", n, sum);
}
}
Sample Output:
Calculate n terms of square natural number and their sum: ----------------------------------------------------------- Input the number of terms : 10 The square natural upto 10 terms are :1 4 9 16 25 36 49 64 81 100 The Sum of Square Natural Number upto 10 terms = 385
Flowchart:
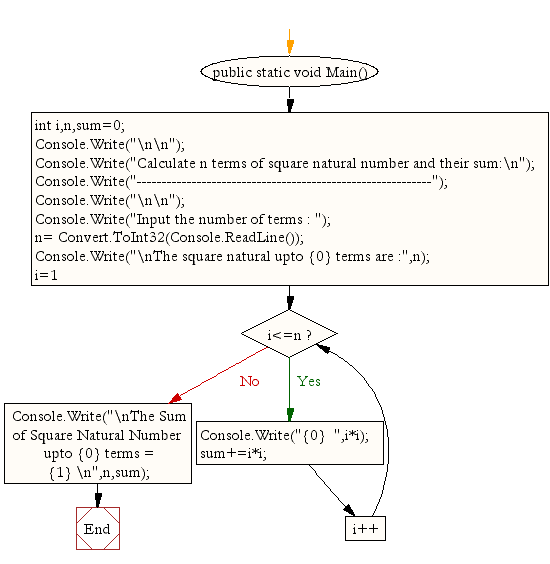
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to find the sum of the series [ x - x^3 + x^5 - x^7 + x^9 -.....].
Next: Write a program in C# Sharp to find the sum of the series 1 +11 + 111 + 1111 + .. n terms.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/csharp-exercises/for-loop/csharp-for-loop-exercise-25.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics