C#: Calculate the sum of the series 1 +11 + 111 + 1111 + .. n terms
Write a program in C# Sharp to find the sum of the series 1 +11 + 111 + 1111 + .. n terms.
Visual Presentation:
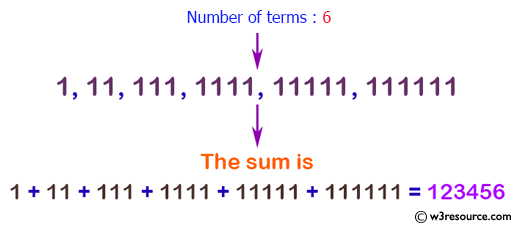
Sample Solution:
C# Sharp Code:
using System; // Importing necessary namespace
public class Exercise26 // Declaration of the Exercise26 class
{
public static void Main() // Main method, entry point of the program
{
int n, i, sum = 0; // Declaration of variables n, i, and sum as integers
int t = 1; // Initializing t as 1
Console.Write("\n\n"); // Displaying new lines
Console.Write("Calculate the sum of the series 1 +11 + 111 + 1111 + .. n terms:\n"); // Displaying the purpose of the program
Console.Write("------------------------------------------------------------------"); // Displaying a separator
Console.Write("\n\n"); // Displaying new lines
Console.Write("Input the number of terms : "); // Prompting the user to input the number of terms
n = Convert.ToInt32(Console.ReadLine()); // Reading the number of terms entered by the user
// Loop to generate the series and calculate its sum
for (i = 1; i <= n; i++)
{
Console.Write("{0} + ", t); // Displaying the current term in the series
sum = sum + t; // Calculating the sum of the series
t = (t * 10) + 1; // Generating the next term in the series
}
Console.Write("\nThe Sum is : {0}\n", sum); // Displaying the total sum of the series
}
}
Sample Output:
Calculate the sum of the series 1 +11 + 111 + 1111 + .. n terms: ------------------------------------------------------------------ Input the numbe of terms : 6 1 + 11 + 111 + 1111 + 11111 + 111111 + The Sum is : 123456
Flowchart:
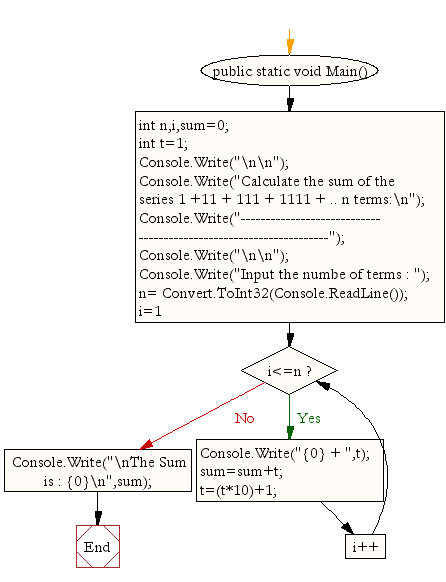
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to display the n terms of square natural number and their sum.
Next: Write a C# Sharp Program to check whether a given number is perfect number or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.