C#: Check whether a given number is perfect number or not
Write a C# Sharp program for checking if a number is a perfect number.
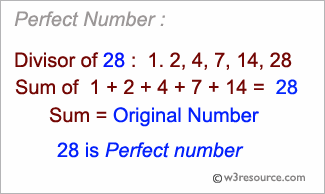
Sample Solution:-
C# Sharp Code:
using System; // Importing necessary namespace
public class Exercise27 // Declaration of the Exercise27 class
{
public static void Main() // Main method, entry point of the program
{
int n, i, sum; // Declaration of variables n, i, and sum as integers
Console.Write("\n\n"); // Displaying new lines
Console.Write("Check whether a given number is a perfect number or not:\n"); // Displaying the purpose of the program
Console.Write("--------------------------------------------------------"); // Displaying a separator
Console.Write("\n\n"); // Displaying new lines
Console.Write("Input the number : "); // Prompting the user to input a number
n = Convert.ToInt32(Console.ReadLine()); // Reading the number entered by the user
sum = 0; // Initializing sum to 0
Console.Write("The positive divisors : "); // Displaying a message
// Loop to find and display the positive divisors of the number
for (i = 1; i < n; i++)
{
if (n % i == 0) // Checking if 'i' is a divisor of 'n'
{
sum = sum + i; // Calculating the sum of divisors
Console.Write("{0} ", i); // Displaying the divisor
}
}
Console.Write("\nThe sum of the divisors is : {0}", sum); // Displaying the sum of divisors
// Checking if the sum of divisors is equal to the original number
if (sum == n)
Console.Write("\nSo, the number is a perfect number."); // Displaying the result if the number is perfect
else
Console.Write("\nSo, the number is not a perfect number."); // Displaying the result if the number is not perfect
Console.Write("\n"); // Displaying a new line
}
}
Sample Output:
Check whether a given number is perfect number or not: -------------------------------------------------------- Input the number : 20 The positive divisor : 1 2 4 5 10 The sum of the divisor is : 22 So, the number is not perfect.
Flowchart:
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to find the sum of the series 1 +11 + 111 + 1111 + .. n terms.
Next: Write a C# Sharp Program to find the perfect numbers within a given range of number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.