C#: Display by Pascal's triangle
Write a C# Sharp program to display Pascal's triangle.
Construction of Pascal's Triangle:
As shown in Pascal's triangle, each element is equal to the sum of the two numbers immediately above it.
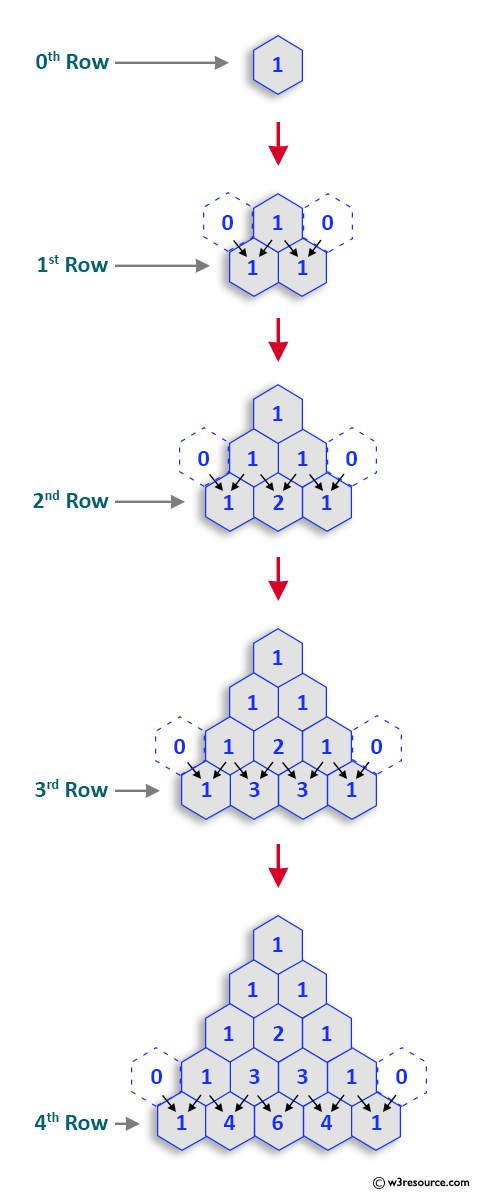
Sample Solution:-
C# Sharp Code:
using System; // Importing necessary namespace
public class Exercise33 // Declaration of the Exercise33 class
{
public static void Main() // Main method, entry point of the program
{
int no_row, c = 1, blk, i, j; // Declaration of variables
Console.Write("\n\n"); // Displaying new lines
Console.Write("Display the Pascal's triangle:\n"); // Displaying the purpose of the program
Console.Write("--------------------------------\n\n"); // Displaying a separator and new lines
Console.Write("Input number of rows: "); // Prompting the user to input the number of rows
no_row = Convert.ToInt32(Console.ReadLine()); // Reading the number entered by the user
// Loop to create and display Pascal's triangle
for (i = 0; i < no_row; i++)
{
for (blk = 1; blk <= no_row - i; blk++)
Console.Write(" "); // Adding spaces for formatting the triangle
for (j = 0; j <= i; j++)
{
if (j == 0 || i == 0)
c = 1; // First and last elements in a row of Pascal's triangle are always 1
else
c = c * (i - j + 1) / j; // Calculating binomial coefficient
Console.Write("{0} ", c); // Displaying the current value of c in the triangle
}
Console.Write("\n"); // Moving to the next line for the new row
}
}
}
Sample Output:
Display the Pascal's triangle: -------------------------------- Input number of rows: 8 1 1 1 1 2 1 1 3 3 1 1 4 6 4 1 1 5 10 10 5 1 1 6 15 20 15 6 1 1 7 21 35 35 21 7 1
Flowchart:
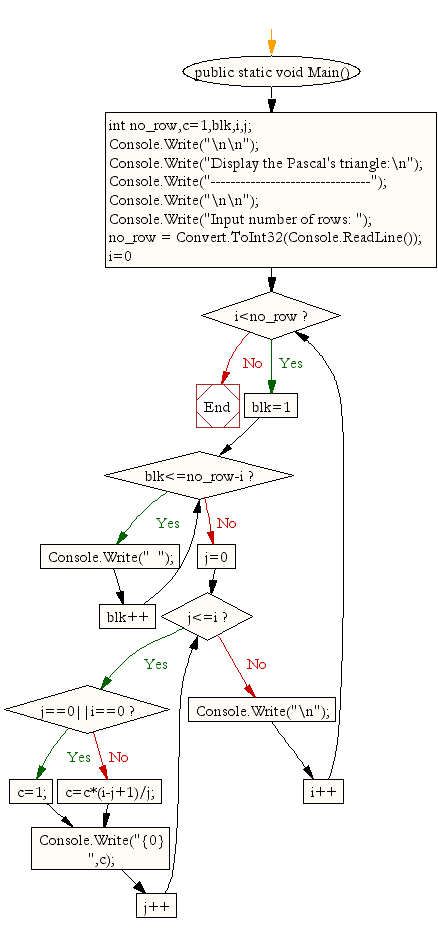
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C# Sharp Program to determine whether a given number is prime or not.
Next: Write a program in C# Sharp to find the prime numbers within a range of numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.