C#: Find the prime numbers within a range of numbers
Write a program in C# Sharp to find prime numbers within a range of numbers.
Visual Presentation:
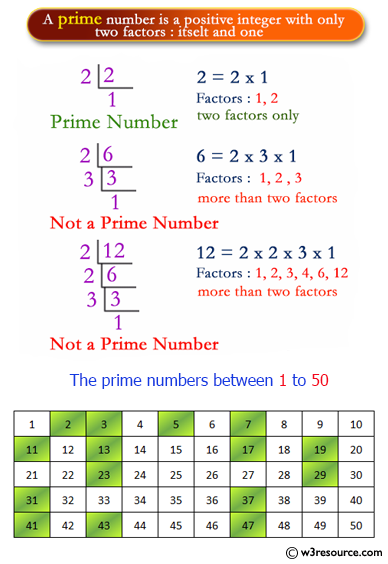
Sample Solution:
C# Sharp Code:
using System; // Importing necessary namespace
public class Exercise34 // Declaration of the Exercise34 class
{
public static void Main() // Main method, entry point of the program
{
int num, i, ctr, stno, enno; // Declaration of variables
Console.Write("\n\n"); // Displaying new lines
Console.Write("Find the prime numbers within a range of numbers:\n"); // Displaying the purpose of the program
Console.Write("---------------------------------------------------\n\n"); // Displaying a separator and new lines
Console.Write("Input starting number of range: "); // Prompting the user to input the starting number of the range
stno = Convert.ToInt32(Console.ReadLine()); // Reading the starting number entered by the user
Console.Write("Input ending number of range : "); // Prompting the user to input the ending number of the range
enno = Convert.ToInt32(Console.ReadLine()); // Reading the ending number entered by the user
Console.Write("The prime numbers between {0} and {1} are : \n", stno, enno); // Displaying the range for which primes are being found
// Loop to find and display prime numbers within the given range
for (num = stno; num <= enno; num++)
{
ctr = 0; // Counter to check for factors of each number
// Loop to check if the number is prime or not
for (i = 2; i <= num / 2; i++)
{
if (num % i == 0)
{
ctr++; // Incrementing the counter if the number has factors other than 1 and itself
break; // Exiting the loop if a factor is found
}
}
// If the counter is 0 and the number is not 1, it's a prime number
if (ctr == 0 && num != 1)
{
Console.Write("{0} ", num); // Displaying the prime number
}
}
Console.Write("\n"); // Moving to the next line for better readability
}
}
Sample Output:
Find the prime numbers within a range of numbers: --------------------------------------------------- Input starting number of range: 1 Input ending number of range : 50 The prime numbers between 1 and 50 are : 2 3 5 7 11 13 17 19 23 29 31 37 41 43 47
Flowchart:
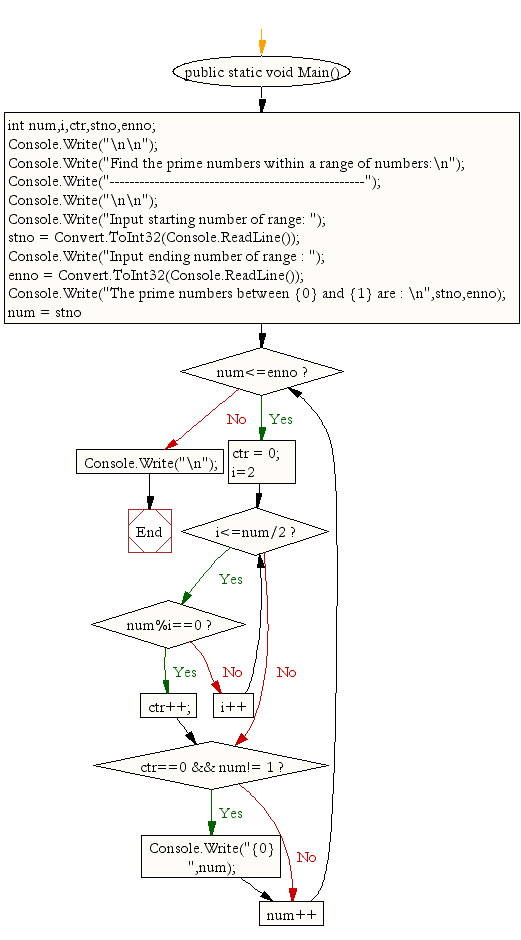
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C# Sharp Program to display by Pascal's triangle
Next: Write a program in C# Sharp to display the first n terms of Fibonacci series.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.