C#: Display the first n terms of Fibonacci series
C# Sharp For Loop: Exercise-35 with Solution
Write a program in C# Sharp to display the first n terms of Fibonacci series.
The series is as follows :
Fibonacci series 0 1 2 3 5 8 13 .....
Visual Presentation:
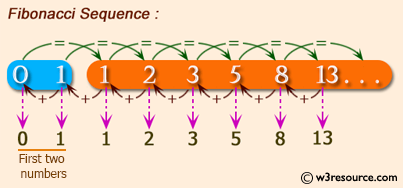
Sample Solution:
C# Sharp Code:
using System; // Importing necessary namespace
public class Exercise35 // Declaration of the Exercise35 class
{
public static void Main() // Main method, entry point of the program
{
int prv = 0, pre = 1, trm, i, n; // Declaration of variables and initializing prv and pre for the first two numbers in the Fibonacci sequence
Console.Write("\n\n"); // Displaying new lines
Console.Write("Display the first n terms of Fibonacci series:\n"); // Displaying the purpose of the program
Console.Write("------------------------------------------------\n\n"); // Displaying a separator and new lines
Console.Write("Input number of terms to be displayed: "); // Prompting the user to input the number of terms to display
n = Convert.ToInt32(Console.ReadLine()); // Reading the number of terms entered by the user
Console.Write("Here is the Fibonacci series up to {0} terms: \n", n); // Displaying the number of terms for the Fibonacci series
Console.Write("{0} {1}", prv, pre); // Displaying the first two terms of the series
// Loop to generate and display the Fibonacci series up to 'n' terms
for (i = 3; i <= n; i++)
{
trm = prv + pre; // Calculating the next term by adding the previous two terms
Console.Write(" {0} ", trm); // Displaying the next term
prv = pre; // Updating the value of prv with the previous term
pre = trm; // Updating the value of pre with the current term
}
Console.Write("\n"); // Moving to the next line for better readability
}
}
Sample Output:
Display the first n terms of fibonacci series: ------------------------------------------------ Input number of terms to be display : 10 Here is the fibonacci series upto to 10 terms : 0 1 1 2 3 5 8 13 21 34
Flowchart:
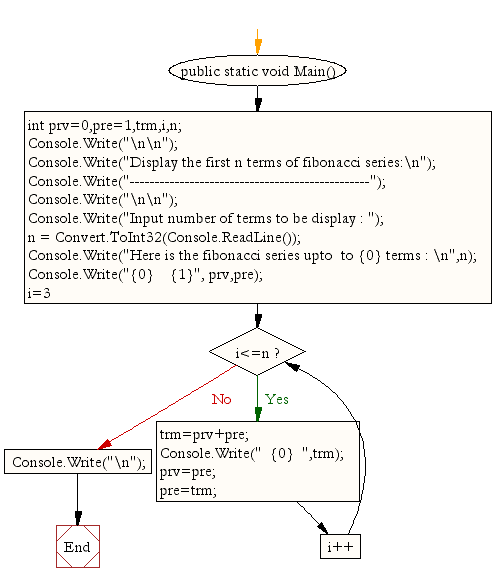
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to find the prime numbers within a range of numbers.
Next: Write a program in C# Sharp to display the such a pattern for n number of rows using a number which will start with the number 1 and the first and a last number of each row will be 1.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/csharp-exercises/for-loop/csharp-for-loop-exercise-35.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics