C#: Generate an Inner Join between two data sets
C# Sharp LINQ : Exercise-25 with Solution
Write a program in C# Sharp to generate an Inner Join between two data sets.
Sample Solution:-
C# Sharp Code:
using System;
using System.Linq;
using System.Collections.Generic;
class LinqExercise25
{
static void Main(string[] args)
{
// Creating a list of Item_mast objects with ItemId and ItemDes properties
List<Item_mast> itemlist = new List<Item_mast>
{
new Item_mast { ItemId = 1, ItemDes = "Biscuit " },
new Item_mast { ItemId = 2, ItemDes = "Chocolate" },
new Item_mast { ItemId = 3, ItemDes = "Butter " },
new Item_mast { ItemId = 4, ItemDes = "Brade " },
new Item_mast { ItemId = 5, ItemDes = "Honey " }
};
// Creating a list of Purchase objects with InvNo, ItemId, and PurQty properties
List<Purchase> purchlist = new List<Purchase>
{
new Purchase { InvNo=100, ItemId = 3, PurQty = 800 },
new Purchase { InvNo=101, ItemId = 2, PurQty = 650 },
new Purchase { InvNo=102, ItemId = 3, PurQty = 900 },
new Purchase { InvNo=103, ItemId = 4, PurQty = 700 },
new Purchase { InvNo=104, ItemId = 3, PurQty = 900 },
new Purchase { InvNo=105, ItemId = 4, PurQty = 650 },
new Purchase { InvNo=106, ItemId = 1, PurQty = 458 }
};
// Printing a prompt for the inner join operation
Console.Write("\nLINQ : Generate an Inner Join between two data sets : ");
Console.Write("\n--------------------------------------------------\n");
Console.Write("Here is the Item_mast List : ");
Console.Write("\n-------------------------\n");
// Displaying the content of the itemlist
foreach (var item in itemlist)
{
Console.WriteLine(
"Item Id: {0}, Description: {1}",
item.ItemId,
item.ItemDes);
}
Console.Write("\nHere is the Purchase List : ");
Console.Write("\n--------------------------\n");
// Displaying the content of the purchlist
foreach (var item in purchlist)
{
Console.WriteLine(
"Invoice No: {0}, Item Id : {1}, Quantity : {2}",
item.InvNo,
item.ItemId,
item.PurQty);
}
Console.Write("\nHere is the list after joining : \n\n");
// Performing an inner join between itemlist and purchlist based on matching ItemId
var innerJoin = from e in itemlist
join d in purchlist on e.ItemId equals d.ItemId
select new
{
itid = e.ItemId,
itdes = e.ItemDes,
prqty = d.PurQty
};
// Displaying the result of the inner join
Console.WriteLine("Item ID\t\tItem Name\tPurchase Quantity");
Console.WriteLine("-------------------------------------------------------");
foreach (var data in innerJoin)
{
Console.WriteLine(data.itid + "\t\t" + data.itdes + "\t\t" + data.prqty);
}
// Waiting for user input before closing the console application
Console.ReadLine();
}
}
// Defining the Item_mast class with ItemId and ItemDes properties
public class Item_mast
{
public int ItemId { get; set; }
public string ItemDes { get; set; }
}
// Defining the Purchase class with InvNo, ItemId, and PurQty properties
public class Purchase
{
public int InvNo { get; set; }
public int ItemId { get; set; }
public int PurQty { get; set; }
}
Sample Output:
LINQ : Generate an Inner Join between two data sets : -------------------------------------------------- Here is the Item_mast List : ------------------------- Item Id: 1, Description: Biscuit Item Id: 2, Description: Chocolate Item Id: 3, Description: Butter Item Id: 4, Description: Brade Item Id: 5, Description: Honey Here is the Purchase List : -------------------------- Invoice No: 100, Item Id : 3, Quantity : 800 Invoice No: 101, Item Id : 2, Quantity : 650 Invoice No: 102, Item Id : 3, Quantity : 900 Invoice No: 103, Item Id : 4, Quantity : 700 Invoice No: 104, Item Id : 3, Quantity : 900 Invoice No: 105, Item Id : 4, Quantity : 650 Invoice No: 106, Item Id : 1, Quantity : 458 Here is the list after joining : Item ID Item Name Purchase Quantity ------------------------------------------------------- 1 Biscuit 458 2 Chocolate 650 3 Butter 800 3 Butter 900 3 Butter 900 4 Brade 700 4 Brade 650
Flowchart:
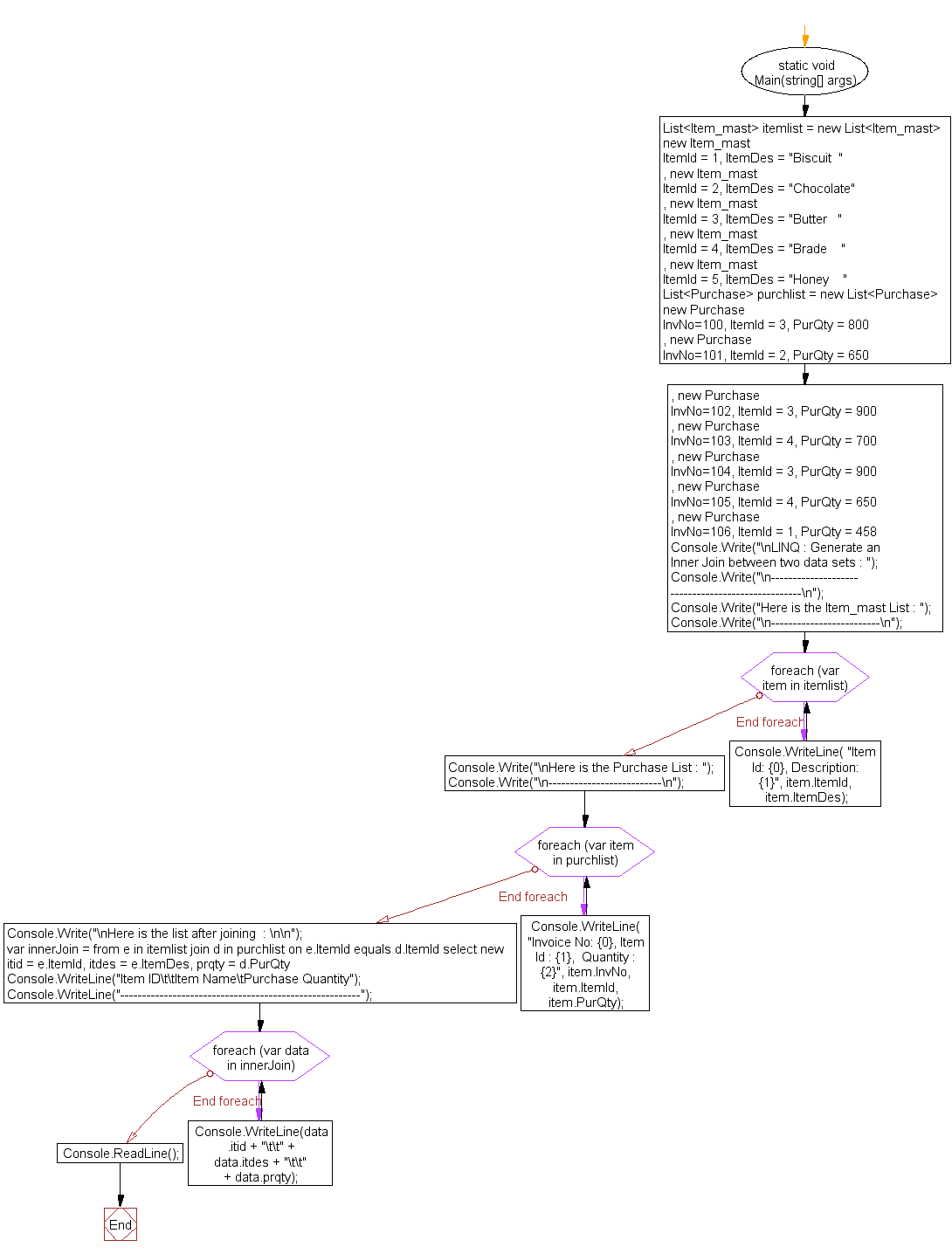
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to generate a Cartesian Product of three sets.
Next: Write a program in C# Sharp to generate a Left Join between two data sets.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/csharp-exercises/linq/csharp-linq-exercise-25.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics