C#: Generate a Left Join between two data sets
Write a program in C# Sharp to generate a Left Join between two data sets.
Sample Solution:
C# Sharp Code:
using System;
using System.Linq;
using System.Collections.Generic;
class LinqExercise26
{
static void Main(string[] args)
{
// Creating a list of Item_mast objects
List<Item_mast> itemlist = new List<Item_mast>
{
// Initializing Item_mast objects with ItemId and ItemDes properties
new Item_mast { ItemId = 1, ItemDes = "Biscuit " },
new Item_mast { ItemId = 2, ItemDes = "Chocolate" },
new Item_mast { ItemId = 3, ItemDes = "Butter " },
new Item_mast { ItemId = 4, ItemDes = "Brade " },
new Item_mast { ItemId = 5, ItemDes = "Honey " }
};
// Creating a list of Purchase objects
List<Purchase> purchlist = new List<Purchase>
{
// Initializing Purchase objects with InvNo, ItemId, and PurQty properties
new Purchase { InvNo=100, ItemId = 3, PurQty = 800 },
new Purchase { InvNo=101, ItemId = 2, PurQty = 650 },
new Purchase { InvNo=102, ItemId = 3, PurQty = 900 },
new Purchase { InvNo=103, ItemId = 4, PurQty = 700 },
new Purchase { InvNo=104, ItemId = 3, PurQty = 900 },
new Purchase { InvNo=105, ItemId = 4, PurQty = 650 },
new Purchase { InvNo=106, ItemId = 1, PurQty = 458 }
};
// Displaying the contents of the Item_mast list
Console.Write("\nLINQ : Generate a Left Join between two data sets : ");
Console.Write("\n--------------------------------------------------\n");
Console.Write("Here is the Item_mast List : ");
Console.Write("\n-------------------------\n");
foreach (var item in itemlist)
{
// Displaying ItemId and ItemDes of each Item_mast object
Console.WriteLine(
"Item Id: {0}, Description: {1}",
item.ItemId,
item.ItemDes);
}
// Displaying the contents of the Purchase list
Console.Write("\nHere is the Purchase List : ");
Console.Write("\n--------------------------\n");
foreach (var item in purchlist)
{
// Displaying InvNo, ItemId, and PurQty of each Purchase object
Console.WriteLine(
"Invoice No: {0}, Item Id : {1}, Quantity : {2}",
item.InvNo,
item.ItemId,
item.PurQty);
}
Console.Write("\nHere is the list after joining : \n\n");
// Performing a left outer join between itemlist and purchlist using LINQ
var leftOuterJoin = from itm in itemlist
join prch in purchlist
on itm.ItemId equals prch.ItemId
into a
from b in a.DefaultIfEmpty(new Purchase())
select new
{
itid = itm.ItemId,
itdes = itm.ItemDes,
prqty = b.PurQty
};
// Displaying the results of the left outer join
Console.WriteLine("Item ID\t\tItem Name\tPurchase Quantity");
Console.WriteLine("-------------------------------------------------------");
foreach (var data in leftOuterJoin)
{
// Displaying ItemId, ItemDes, and PurQty after the left outer join operation
Console.WriteLine(data.itid + "\t\t" + data.itdes + "\t\t" + data.prqty);
}
Console.ReadLine();
}
}
// Class representing the Item_mast object
public class Item_mast
{
public int ItemId { get; set; } // Property for Item ID
public string ItemDes { get; set; } // Property for Item Description
}
// Class representing the Purchase object
public class Purchase
{
public int InvNo { get; set; } // Property for Invoice Number
public int ItemId { get; set; } // Property for Item ID
public int PurQty { get; set; } // Property for Purchase Quantity
}
Sample Output:
LINQ : Generate a Left Join between two data sets : -------------------------------------------------- Here is the Item_mast List : ------------------------- Item Id: 1, Description: Biscuit Item Id: 2, Description: Chocolate Item Id: 3, Description: Butter Item Id: 4, Description: Brade Item Id: 5, Description: Honey Here is the Purchase List : -------------------------- Invoice No: 100, Item Id : 3, Quantity : 800 Invoice No: 101, Item Id : 2, Quantity : 650 Invoice No: 102, Item Id : 3, Quantity : 900 Invoice No: 103, Item Id : 4, Quantity : 700 Invoice No: 104, Item Id : 3, Quantity : 900 Invoice No: 105, Item Id : 4, Quantity : 650 Invoice No: 106, Item Id : 1, Quantity : 458 Here is the list after joining : Item ID Item Name Purchase Quantity ------------------------------------------------------- 1 Biscuit 458 2 Chocolate 650 3 Butter 800 3 Butter 900 3 Butter 900 4 Brade 700 4 Brade 650 5 Honey 0
Flowchart:
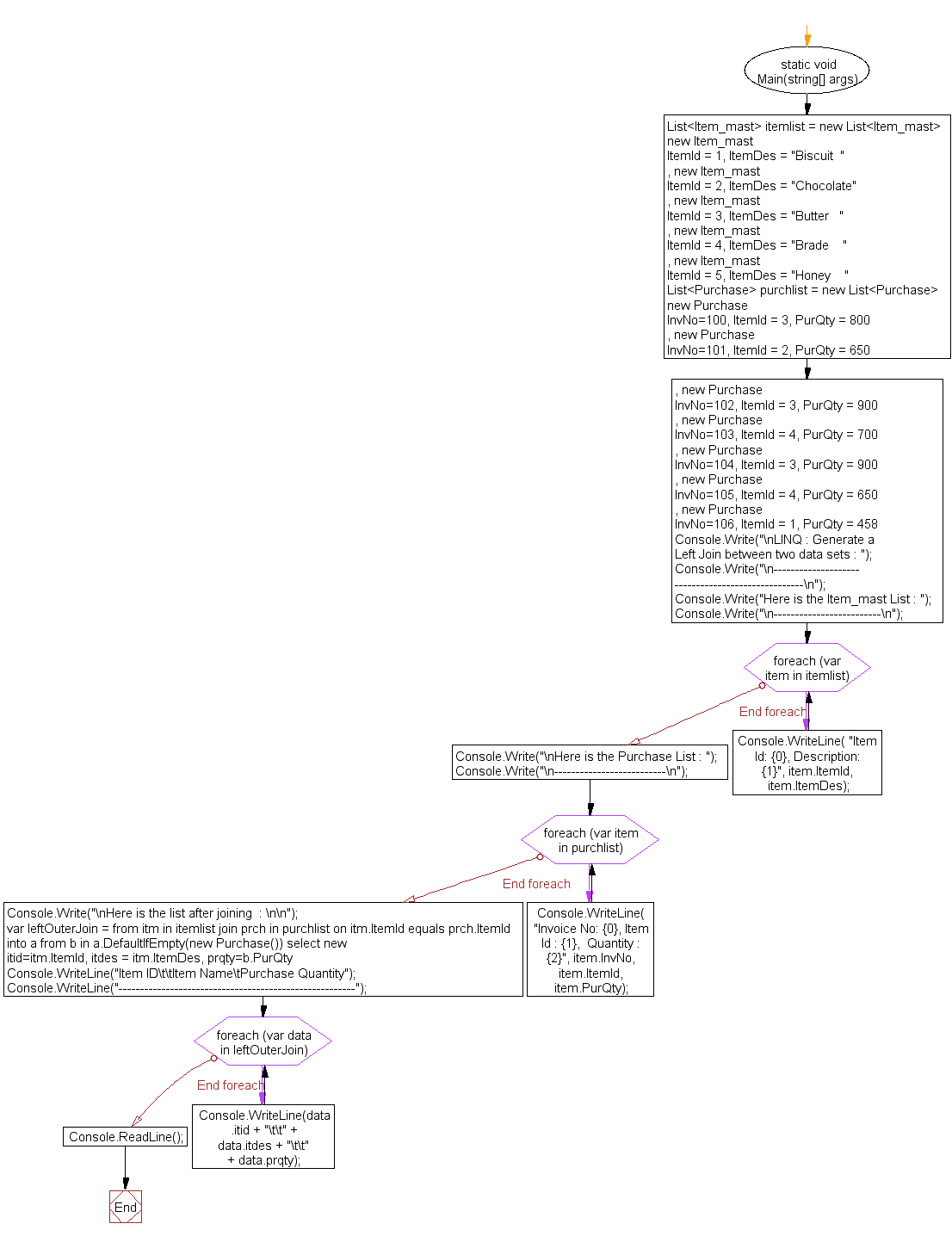
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to generate an Inner Join between two data sets.
Next: Write a program in C# Sharp to generate a Right Outer Join between two data sets.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.