C#: Check whether a given integer is a palindrome integer or not
Write a C# Sharp program to check whether a given integer is a palindrome integer or not. Return true if the number is a palindrome, otherwise return false.
A palindromic number (also known as a numeral palindrome or a numeric palindrome) is a number (such as 16461) that remains the same when its digits are reversed. In other words, it has reflectional symmetry across a vertical axis. The term palindromic is derived from palindrome, which refers to a word (such as rotor or racecar) whose spelling is unchanged when its letters are reversed. The first 30 palindromic numbers (in decimal) are:
0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 11, 22, 33, 44, 55, 66, 77, 88, 99, 101, 111, 121, 131, 141, 151, 161, 171, 181, 191, 202, …
Sample Solution:
C# Sharp Code:
using System;
namespace exercises {
class Program {
static void Main(string[] args) {
int n;
n = 123456;
Console.WriteLine("Original integer value: " + n);
Console.WriteLine("Check the said number is a palindrome number or not:");
Console.WriteLine(test_is_Palindrome(n));
n = 16461;
Console.WriteLine("Original integer value: " + n);
Console.WriteLine("Check the said number is a palindrome number or not:");
Console.WriteLine(test_is_Palindrome(n));
n = -121;
Console.WriteLine("Original integer value: " + n);
Console.WriteLine("Check the said number is a palindrome number or not:");
Console.WriteLine(test_is_Palindrome(n));
}
public static bool test_is_Palindrome(int a)
{
if (a < 0) { return false; }
if (a < 10) { return true; }
var temp = a;
var b = 0;
var digit = 0;
while (temp != 0)
{
digit = temp % 10;
b = b * 10 + digit;
temp /= 10;
}
return a == b;
}
}
}
Sample Output:
Original integer value: 123456 Check the said number is a palindrome number or not: False Original integer value: 16461 Check the said number is a palindrome number or not: True Original integer value: -121 Check the said number is a palindrome number or not: False
Flowchart:
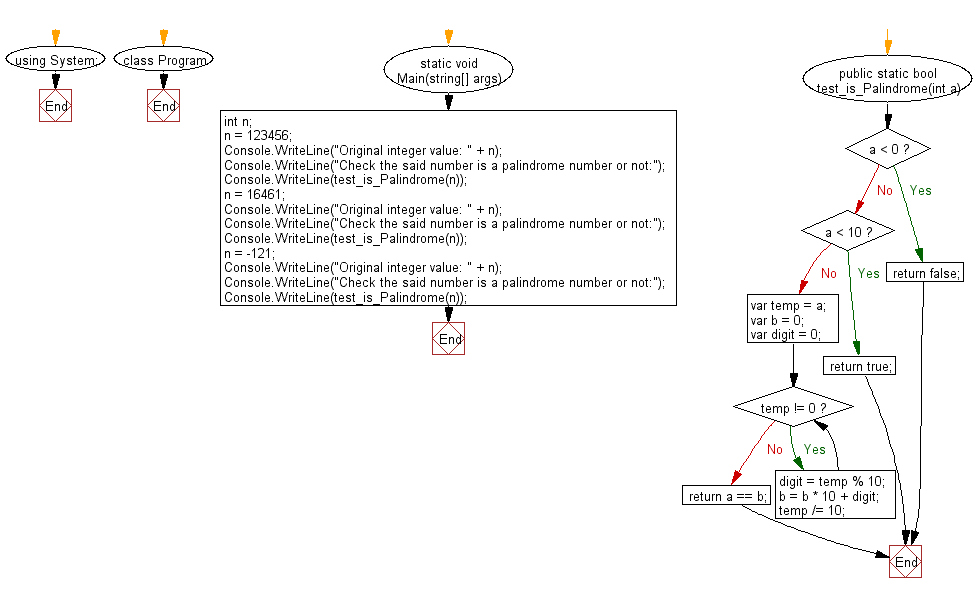
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to convert a given string value to a 32-bit signed integer.
Next: Write a C# Sharp program to convert a given integer value to Roman numerals.What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.