C#: Convert a given integer value to Roman numerals
Write a C# Sharp program to convert a given integer value to Roman numerals.
From Wikipedia,
Roman numerals are a numeral system that originated in ancient Rome and remained the usual way of writing numbers throughout Europe well into the Late Middle Ages. Numbers in this system are represented by combinations of letters from the Latin alphabet. Modern usage employs seven symbols, each with a fixed integer value.
Symbol I V X L C D M Value 1 5 10 50 100 500 1000
Sample Solution:
C# Sharp Code:
using System;
using System.Text;
namespace exercises {
class Program {
static void Main(string[] args) {
int n;
n = 2365;
Console.WriteLine("Original integer value: " + n);
Console.WriteLine("Roman numerals of the said integer value:");
Console.WriteLine(int_to_Roman(n));
n = 254;
Console.WriteLine("Original integer value: " + n);
Console.WriteLine("Roman numerals of the said integer value:");
Console.WriteLine(int_to_Roman(n));
n = 45;
Console.WriteLine("Original integer value: " + n);
Console.WriteLine("Roman numerals of the said integer value:");
Console.WriteLine(int_to_Roman(n));
n = 8;
Console.WriteLine("Original integer value: " + n);
Console.WriteLine("Roman numerals of the said integer value:");
Console.WriteLine(int_to_Roman(n));
}
public static string int_to_Roman(int n)
{
string[] roman_symbol = { "MMM", "MM", "M", "CM", "DCCC", "DCC", "DC", "D", "CD", "CCC", "CC", "C", "XC", "LXXX", "LXX", "LX", "L", "XL", "XXX", "XX", "X", "IX", "VIII", "VII", "VI", "V", "IV", "III", "II", "I" };
int[] int_value = { 3000, 2000, 1000, 900, 800, 700, 600, 500, 400, 300, 200, 100, 90, 80, 70, 60, 50, 40, 30, 20, 10, 9, 8, 7, 6, 5, 4, 3, 2, 1 };
var roman_numerals = new StringBuilder();
var index_num = 0;
while (n != 0)
{
if (n >= int_value[index_num])
{
n -= int_value[index_num];
roman_numerals.Append(roman_symbol[index_num]);
}
else
{
index_num++;
}
}
return roman_numerals.ToString();
}
}
}
Sample Output:
Original integer value: 2365 Roman numerals of the said integer value: MMCCCLXV Original integer value: 254 Roman numerals of the said integer value: CCLIV Original integer value: 45 Roman numerals of the said integer value: XLV Original integer value: 8 Roman numerals of the said integer value: VIII
Flowchart:
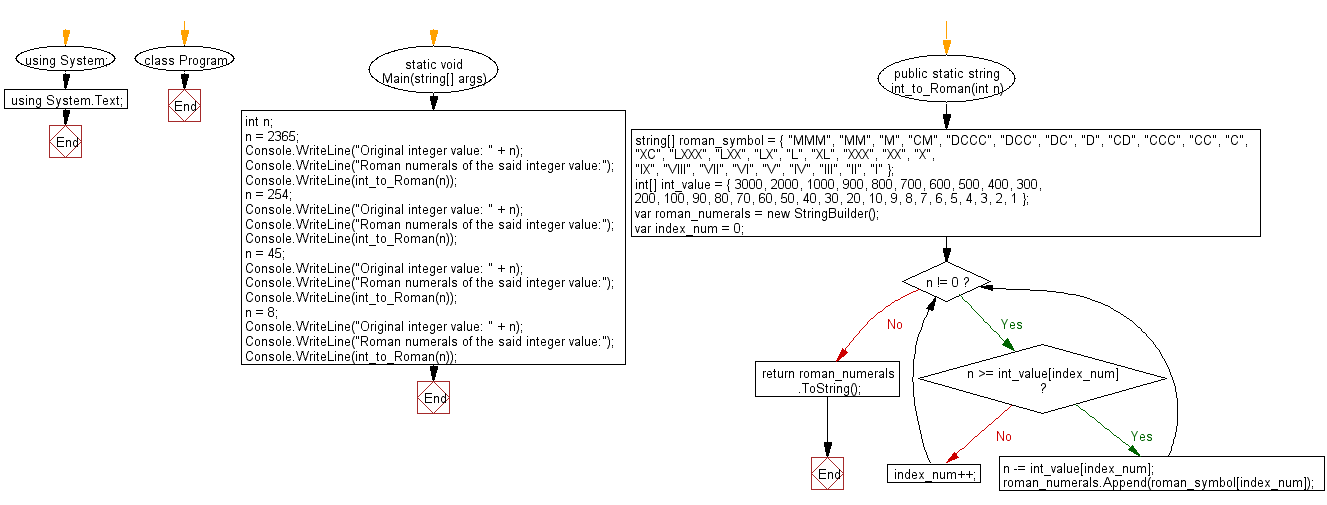
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Next: Write a C# Sharp program to calculate the largest integral value less than or equal and smallest integral value greater than or equal to a given number.What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.