C#: Calculate the largest integral value less than or equal and smallest integral value greater than or equal to a given number
C# Sharp Math: Exercise-14 with Solution
Write a C# Sharp program to calculate the largest integral value less than or equal to and the smallest integral value greater than or equal to a given number.
Sample Solution:
C# Sharp Code:
using System;
using System.Text;
namespace exercises {
class Program {
public static void Main() {
decimal[] values = {
8.03m,
8.34m,
0.12m,
-0.14m,
-8.1m,
-8.6m
};
Console.WriteLine(" Value largest int value smallest int value");
Console.WriteLine(" Value less than or equal greater than or equal");
foreach(decimal value in values)
Console.WriteLine("{0,7} {1,16} {2,20}",
value, Math.Ceiling(value), Math.Floor(value));
}
}
}
Sample Output:
Value largest int value smallest int value Value less than or equal greater than or equal 8.03 9 8 8.34 9 8 0.12 1 0 -0.14 0 -1 -8.1 -8 -9 -8.6 -8 -9
Flowchart:
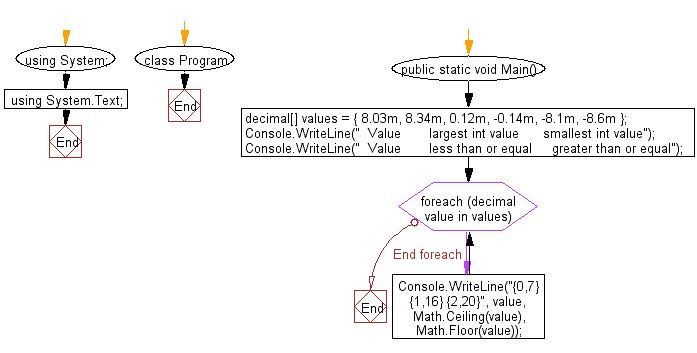
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to convert a given integer value to Roman numerals.
Next: Write a C# Sharp program to convert a given Roman numerals value to an integer value.What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/csharp-exercises/math/csharp-math-exercise-14.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics