C#: Convert a given Roman numerals value to an integer value
C# Sharp Math: Exercise-15 with Solution
Write a C# Sharp program to convert a given Roman numeral value to an integer value.
From Wikipedia,
Roman numerals are a numeral system that originated in ancient Rome and remained the usual way of writing numbers throughout Europe well into the Late Middle Ages. Numbers in this system are represented by combinations of letters from the Latin alphabet. Modern usage employs seven symbols, each with a fixed integer value.
Symbol I V X L C D M Value 1 5 10 50 100 500 1000
Sample Solution:
C# Sharp Code:
using System;
using System.Text;
namespace exercises {
class Program {
static void Main(string[] args) {
string s;
s = "MMCCCLXV";
Console.WriteLine("Original integer value: " +s);
Console.WriteLine("Integer value of the said Roman numerals:");
Console.WriteLine(roman_to_int(s));
s = "CCLIV";
Console.WriteLine("Original integer value: " + s);
Console.WriteLine("Integer value of the said Roman numerals:");
Console.WriteLine(roman_to_int(s));
s = "XLV";
Console.WriteLine("Original integer value: " + s);
Console.WriteLine("Integer value of the said Roman numerals:");
Console.WriteLine(roman_to_int(s));
s = "VIII";
Console.WriteLine("Original integer value: " + s);
Console.WriteLine("Integer value of the said Roman numerals:");
Console.WriteLine(roman_to_int(s));
}
public static int roman_to_int(string str1)
{
var num = 0;
for (int i = 0; i < str1.Length; i++)
{
if (i > 0 && find_value(str1[i]) > find_value(str1[i - 1]))
{
num += find_value(str1[i]) - find_value(str1[i - 1]) * 2;
}
else
{
num += find_value(str1[i]);
}
}
return num;
}
public static int find_value(char chr)
{
switch (chr)
{
case 'I': return 1;
case 'V': return 5;
case 'X': return 10;
case 'L': return 50;
case 'C': return 100;
case 'D': return 500;
case 'M': return 1000;
default: return 0;
}
}
}
}
Sample Output:
Original integer value: MMCCCLXV Integer value of the said Roman numerals: 2365 Original integer value: CCLIV Integer value of the said Roman numerals: 254 Original integer value: XLV Integer value of the said Roman numerals: 45 Original integer value: VIII Integer value of the said Roman numerals: 8
Flowchart:
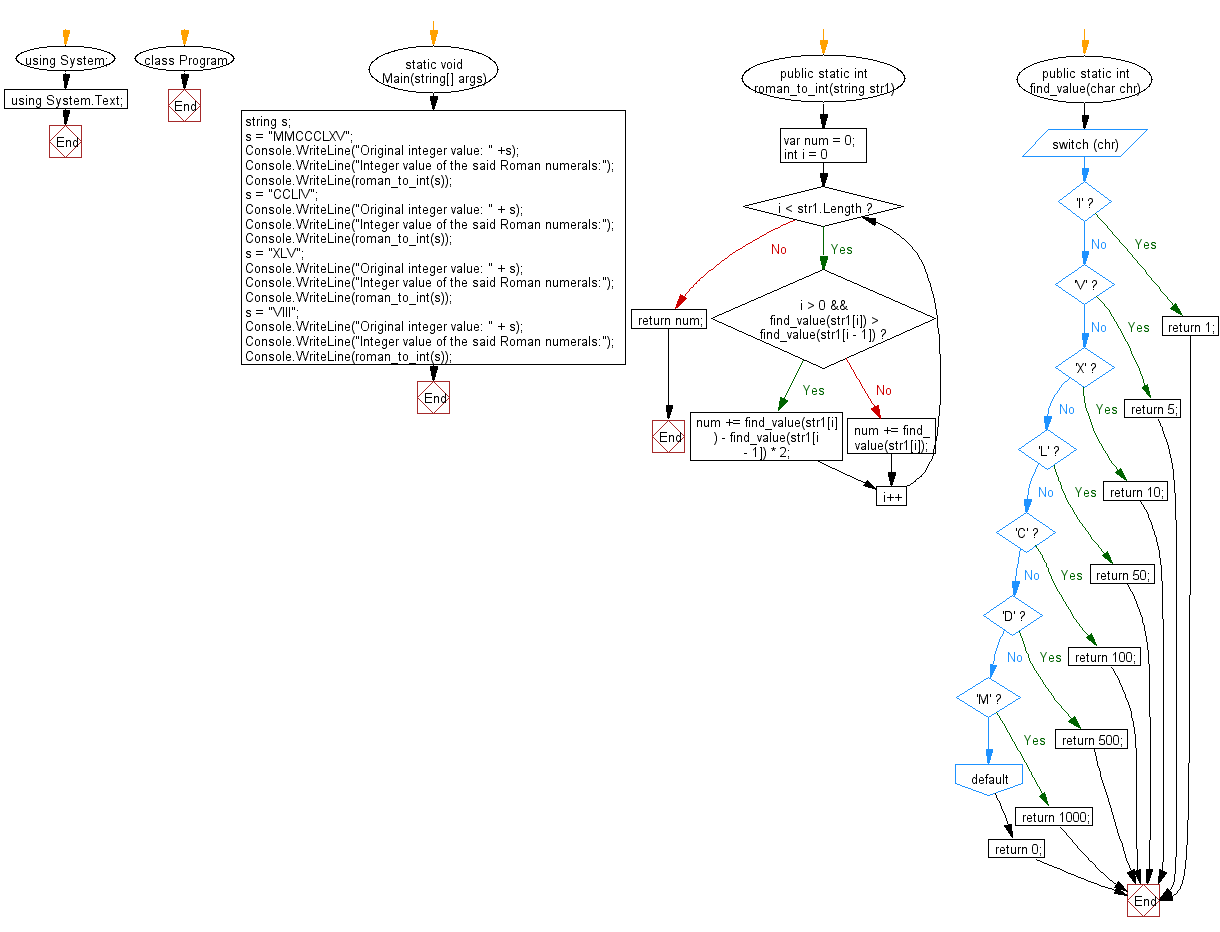
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Next: Write a C# program to divide two given integers (dividend and divisor) and get the quotient without using multiplication, division and mod operator.What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/csharp-exercises/math/csharp-math-exercise-15.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics