C#: Divide two given integers and get the quotient without using multiplication, division and mod operator
Write a C# program to divide two given integers (dividend and divisor) and get the quotient without using multiplication, division and the mod operator.
Sample Solution:
C# Sharp Code:
using System;
using System.Text;
namespace exercises {
class Program {
static void Main(string[] args) {
int dividend, divisor;
dividend = 7;
divisor = 3;
if (divisor > 0) {
Console.WriteLine("Dividend = " + dividend + ", Divisor = " + divisor);
Console.WriteLine("Quotient= " + Divide(dividend, divisor));
}
dividend = -17;
divisor = 5;
if (divisor > 0) {
Console.WriteLine("Dividend = " + dividend + ", Divisor = " + divisor);
Console.WriteLine("Quotient= " + Divide(dividend, divisor));
}
dividend = 35;
divisor = 7;
if (divisor > 0) {
Console.WriteLine("Dividend = " + dividend + ", Divisor = " + divisor);
Console.WriteLine("Quotient= " + Divide(dividend, divisor));
}
}
public static int Divide(int dividend, int divisor) {
uint x = dividend > 0 ? (uint) dividend : (uint) - dividend;
uint y = divisor > 0 ? (uint) divisor : (uint) - divisor;
uint result = 0, z = 0;
var idx = 0;
while (x >= y) {
z = y;
for (idx = 0; x >= z && z != 0; idx++, z *= 2) {
x -= z;
result += (uint) 1 << idx;
}
}
return (dividend ^ divisor) >> 31 == -1 ?
(int) - result :
result > int.MaxValue ? int.MaxValue : (int) result;
}
}
}
Sample Output:
Dividend = 7, Divisor = 3 Quotient= 2 Dividend = -17, Divisor = 5 Quotient= -3 Dividend = 35, Divisor = 7 Quotient= 5
Flowchart:
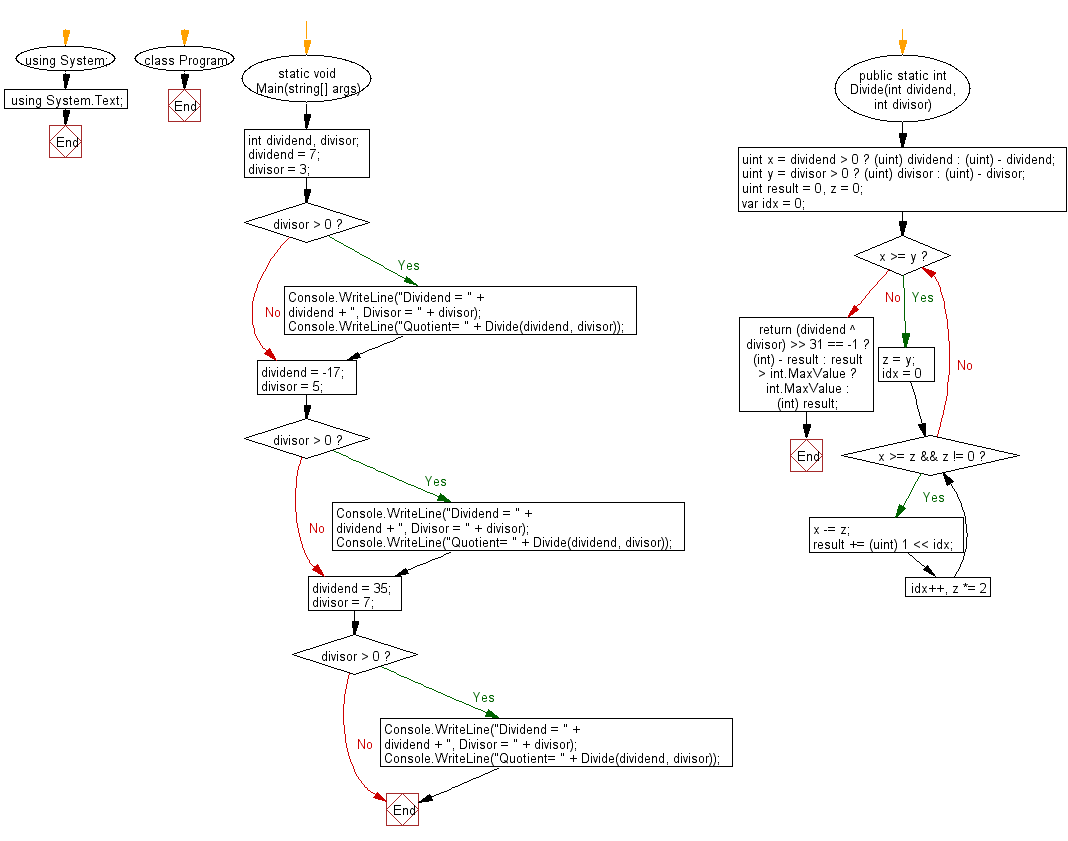
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to convert a given Roman numerals value to an integer value.
Next: Write a C# program to given two non-negative integers n1 and n2 represented as string, return the string represented product of n1 and n2.What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.