C#: Create string represented product of two non-negative integers
C# Sharp Math: Exercise-17 with Solution
Write a C# program to return the string representation of the product of two non-negative integers n1 and n2, given two non-negative integers n1 and n2.
Sample Solution:
C# Sharp Code:
using System;
using System.Text;
namespace exercises {
class Program {
static void Main(string[] args) {
string ns1, ns2;
ns1 = "12";
ns2 = "5";
Console.WriteLine("Non-negative integer1(string) = " + ns1 + ", Non-negative integer2(string) = " + ns2);
Console.WriteLine("Product = "+ str_num_multiply(ns1, ns2));
ns1 = "221";
ns2 = "415";
Console.WriteLine("Non-negative integer1(string) = " + ns1 + ", Non-negative integer2(string) = " + ns2);
Console.WriteLine("Product = "+ str_num_multiply(ns1, ns2));
ns1 = "0";
ns2 = "15";
Console.WriteLine("Non-negative integer1(string) = " + ns1 + ", Non-negative integer2(string) = " + ns2);
Console.WriteLine("Product = "+ str_num_multiply(ns1, ns2));
}
public static string str_num_multiply(string ns1, string ns2)
{
if (ns1 == "0" || ns2 == "0") { return "0"; }
var max_pos_num = 1000000000;
var max_pos_num_len = 9;
var num1Value = new long[ns1.Length / max_pos_num_len + 1];
var num2Value = new long[ns2.Length / max_pos_num_len + 1];
int i, j, temp, num, idx = 0;
for (i = ns1.Length; i > 0; i -= max_pos_num_len)
{
num = 0;
temp = i > max_pos_num_len ? i - max_pos_num_len : 0;
for (j = temp; j < i; j++)
{
num = num * 10 + (ns1[j] - '0');
}
num1Value[idx++] = num;
}
idx = 0;
for (i = ns2.Length; i > 0; i -= max_pos_num_len)
{
num = 0;
temp = i > max_pos_num_len ? i - max_pos_num_len : 0;
for (j = temp; j < i; j++)
{
num = num * 10 + (ns2[j] - '0');
}
num2Value[idx++] = num;
}
var result_val = new long[num1Value.Length + num2Value.Length];
for (i = 0; i < num1Value.Length; i++)
{
for (j = 0; j < num2Value.Length; j++)
{
result_val[i + j] += num1Value[i] * num2Value[j];
if (result_val[i + j] >= max_pos_num)
{
result_val[i + j + 1] += result_val[i + j] / max_pos_num;
result_val[i + j] = result_val[i + j] % max_pos_num;
}
}
}
var result = new StringBuilder();
var tempStr = string.Empty;
var flag = false;
for (i = result_val.Length - 1; i >= 0; i--)
{
if (result_val[i] != 0)
{
tempStr = result_val[i].ToString();
if (flag)
{
result.Append('0', 9 - tempStr.Length);
}
result.Append(result_val[i].ToString());
flag = true;
}
}
return result.ToString();
}
}
}
Sample Output:
Non-negative integer1(string) = 12, Non-negative integer2(string) = 5 Product = 60 Non-negative integer1(string) = 221, Non-negative integer2(string) = 415 Product = 91715 Non-negative integer1(string) = 0, Non-negative integer2(string) = 15 Product = 0
Flowchart:
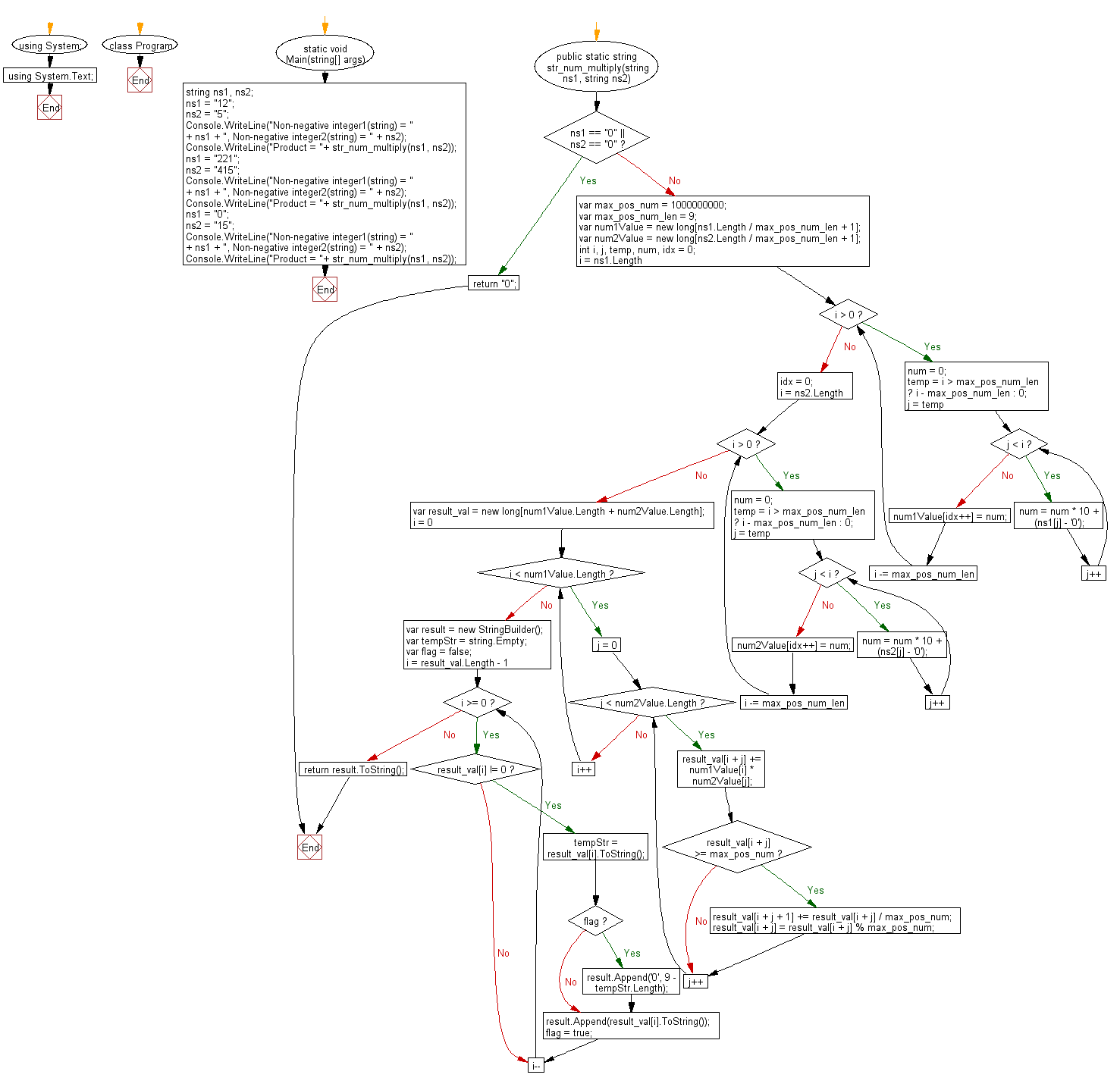
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# program to divide two given integers (dividend and divisor) and get the quotient without using multiplication, division and mod operator. Next: Write a C# Sharp program to compute the sum of the positive and negative numbers of an array of integers and display the largest sum.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics