C#: Compute the sum of the positive and negative numbers of an array of integers and display the largest sum
Write a C# Sharp program to compute the sum of the positive and negative numbers of an array of integers and display the largest sum.
Sample Solution:
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
int[] nums = { -10, -11, -12, -13, -14, 15, 16, 17, 18, 19, 20 };
Console.WriteLine("Original array elements:");
for (int i = 0; i < nums.Length; i++)
{
Console.Write(nums[i] + " ");
}
Console.WriteLine("\nLargest sum - Positive/Negative numbers of the said array: " + test(nums));
int[] nums1 = { -11, -22, -44, 0, 3, 4 , 5, 9 };
Console.WriteLine("\nOriginal array elements:");
for (int i = 0; i < nums1.Length; i++)
{
Console.Write(nums1[i] + " ");
}
Console.WriteLine("\nLargest sum - Positive/Negative numbers of the said array: " + test(nums1));
}
public static int test(int[] nums)
{
int[] sums = { nums.Where(x => x > 0).Sum(),nums.Where(x => x < 0).Sum() };
return sums.OrderByDescending(x => Math.Abs(x)).First();
}
}
}
Sample Output:
Original array elements: -10 -11 -12 -13 -14 15 16 17 18 19 20 Largest sum - Positive/Negative numbers of the said array: 105 Original array elements: -11 -22 -44 0 3 4 5 9 Largest sum - Positive/Negative numbers of the said array: -77
Flowchart:
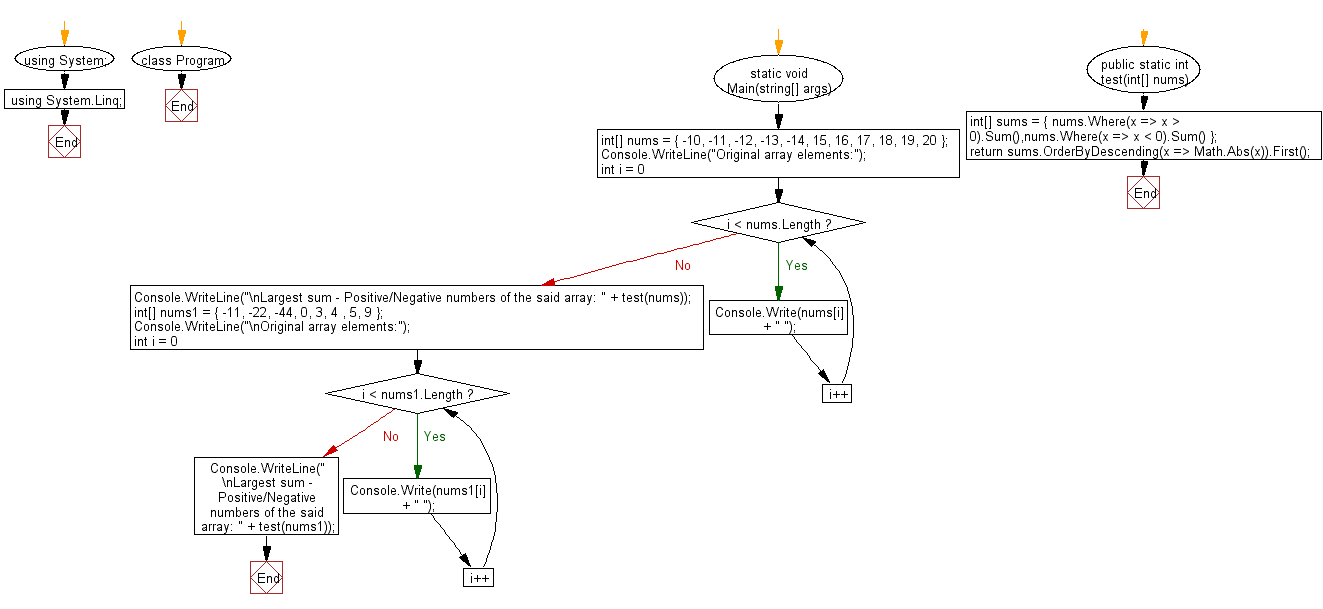
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# program to given two non-negative integers n1 and n2 represented as string, return the string represented product of n1 and n2. Next: Write a C# Sharp program to find the value of PI up to n (given number) decimals places.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.