C#: Sort a given positive number in descending/ascending order
Write a C# Sharp program to sort a given positive number in descending/ascending order.
Descending -> Highest to lowest.
Ascending -> Lowest to highest.
Sample Solution:
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
int n = 134543;
Console.WriteLine("Original Number: " + n);
Console.WriteLine("\nDescending order of the said number: " + test_dsc(n));
Console.WriteLine("\nAscending order of the said number: " + test_asc(n));
n = 4375973;
Console.WriteLine("\nOriginal Number: " + n);
Console.WriteLine("\nDescending order of the said number: " + test_dsc(n));
Console.WriteLine("\nAscending order of the said number: " + test_asc(n));
}
public static int test_dsc(int num)
{
return Convert.ToInt32(String.Concat(num.ToString().OrderByDescending(x => x)));
}
public static int test_asc(int num)
{
return Convert.ToInt32(String.Concat(num.ToString().OrderBy(x => x)));
}
}
}
Sample Output:
Original Number: 134543 Descending order of the said number: 544331 Ascending order of the said number: 133445 Original Number: 4375973 Descending order of the said number: 9775433 Ascending order of the said number: 3345779
Flowchart:
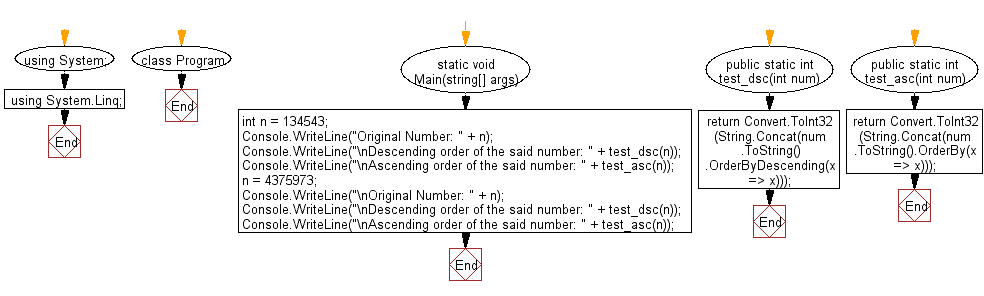
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to get the nth tetrahedral number from a given integer(n) value.
Next: Write a C# Sharp program to check whether a given number (integer) is Oddish or Evenish.What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.