C#: Check whether a given number (integer) is Oddish or Evenish
C# Sharp Math: Exercise-23 with Solution
Write a C# Sharp program to check whether a given number (integer) is oddish or evenish.
A number is called "Oddish" if the sum of all of its digits is odd, and a number is called "Evenish" if the sum of all of its digits is even.
Example:
120 -> Oddish
321 -> Evenish
43 -> Oddish
4433 -> Evenish
373 -> Oddish
Sample Solution:
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
int n = 120;
Console.WriteLine("Original number: " + n);
Console.WriteLine("Check the said number is Oddish or Evenish! " + test(n));
n = 321;
Console.WriteLine("\nOriginal number: " + n);
Console.WriteLine("Check the said number is Oddish or Evenish! " + test(n));
n = 43;
Console.WriteLine("\nOriginal number: " + n);
Console.WriteLine("Check the said number is Oddish or Evenish! " + test(n));
n = 4433;
Console.WriteLine("\nOriginal number: " + n);
Console.WriteLine("Check the said number is Oddish or Evenish! " + test(n));
}
public static string test(int n)
{
string str = n.ToString();
int total = 0;
for (int i = 0; i < str.Length; i++)
total += int.Parse(str[i].ToString());
return (total % 2 == 0 ? "Evenish" : "Oddish");
}
}
}
Sample Output:
Original number: 120 Check the said number is Oddish or Evenish! Oddish Original number: 321 Check the said number is Oddish or Evenish! Evenish Original number: 43 Check the said number is Oddish or Evenish! Oddish Original number: 4433 Check the said number is Oddish or Evenish! Evenish
Flowchart:
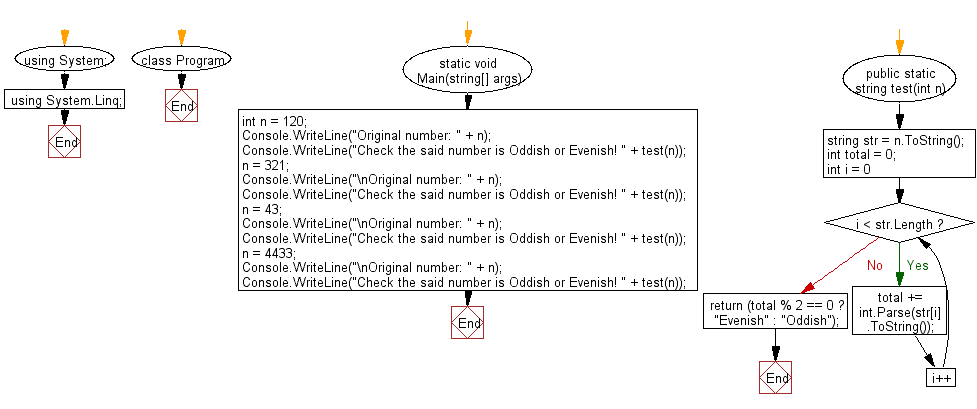
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to sort a given positive number in descending/ascending order.
Next: Write a C# Sharp program to reverse the binary representation of an given integer and convert the reversed binary number into an integer.What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/csharp-exercises/math/csharp-math-exercise-23.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics