C#: Reverse the binary representation of an integer and convert it into an integer
Write a C# Sharp program to reverse the binary representation of a given number and convert the reversed binary number into an integer.
Sample Solution:
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
int n = 120;
Console.WriteLine("Original number: " + n);
Console.WriteLine("Reverse the binary representation of the said integer and convert it into an integer: " + test(n));
n = 321;
Console.WriteLine("\nOriginal number: " + n);
Console.WriteLine("Reverse the binary representation of the said integer and convert it into an integer: " + test(n));
n = 43;
Console.WriteLine("\nOriginal number: " + n);
Console.WriteLine("Reverse the binary representation of the said integer and convert it into an integer: " + test(n));
n = 4433;
Console.WriteLine("\nOriginal number: " + n);
Console.WriteLine("Reverse the binary representation of the said integer and convert it into an integer: " + test(n));
}
public static int test(int n)
{
return (Convert.ToInt32(new string(Convert.ToString(n, 2).Reverse().ToArray()), 2));
}
}
}
Sample Output:
Original number: 120 Reverse the binary representation of the said integer and convert it into an integer: 15 Original number: 321 Reverse the binary representation of the said integer and convert it into an integer: 261 Original number: 43 Reverse the binary representation of the said integer and convert it into an integer: 53 Original number: 4433 Reverse the binary representation of the said integer and convert it into an integer: 4433
Flowchart:
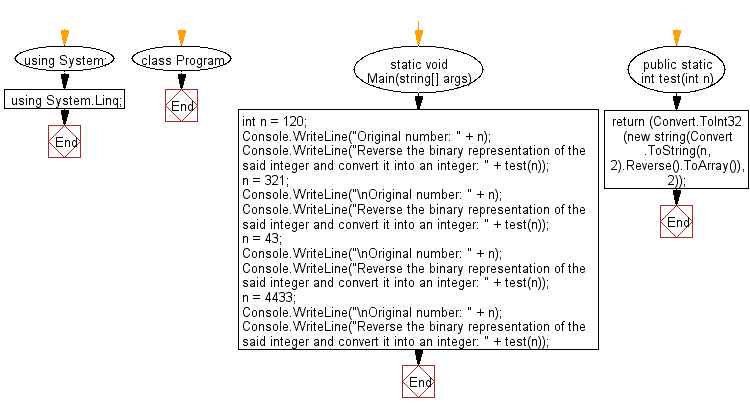
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to check whether a given number (integer) is Oddish or Evenish.
Next: Closest Palindrome Number.What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.