C#: Check a number is uban number or not
From numbersaplenty -
A number nis called uban if its name (in English) does not contain the letter "u".
In particular, it cannot contain the terms "four", "hundred", and "thousand", So the uban number following 99 is 1000000.
Here are some uban numbers:
The first 600 uban numbers :
1, 2, 3, 5, 6, 7, 8, 9, 10, 11, 12, 13, 15, 16, 17, 18, 19, 20, 21, 22, 23, 25, 26, 27, 28, 29, 30, 31, 32, 33, 35, 36, 37, 38, 39, 40, 41, 42, 43, 45, 46, 47, 48, 49, 50, 51, 52, 53, 55, 56, 57, 58, 59, 60, 61, 62, 63, 65, 66, 67, 68, 69, 70, 71, 72, 73, 75, 76, 77, 78, 79, 80, 81, 82, 83, 85, 86, 87, 88, 89, 90, 91, 92, 93, 95, 96, 97, 98, 99, 1000000, 1000001, 1000002, 1000003, 1000005, 1000006,....
Write a C# Sharp program that takes an integer and determines whether it is uban or not.
Sample Data:
(63) -> True
(100) -> False
(100000) -> False
(1000005) -> True
Sample Solution:
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
int n = 63;
Console.WriteLine("Original Number: " + n);
Console.WriteLine("Check the said number is uban number or not: " + test(n));
n = 100;
Console.WriteLine("Original Number: " + n);
Console.WriteLine("Check the said number is uban number or not: " + test(n));
n = 100000;
Console.WriteLine("Original Number: " + n);
Console.WriteLine("Check the said number is uban number or not: " + test(n));
n = 1000005;
Console.WriteLine("Original Number: " + n);
Console.WriteLine("Check the said number is uban number or not: " + test(n));
}
public static bool test(int n)
{
if (n > 999 && n <= 999999)
{
return false;
}
else if (n > 999999)
{
return true;
}
else if (n > 99)
{
return false;
}
else
{
return n % 10 != 4;
}
}
}
}
Sample Output:
Original Number: 63 Check the said number is uban number or not: True Original Number: 100 Check the said number is uban number or not: False Original Number: 100000 Check the said number is uban number or not: False Original Number: 1000005 Check the said number is uban number or not: True
Flowchart:
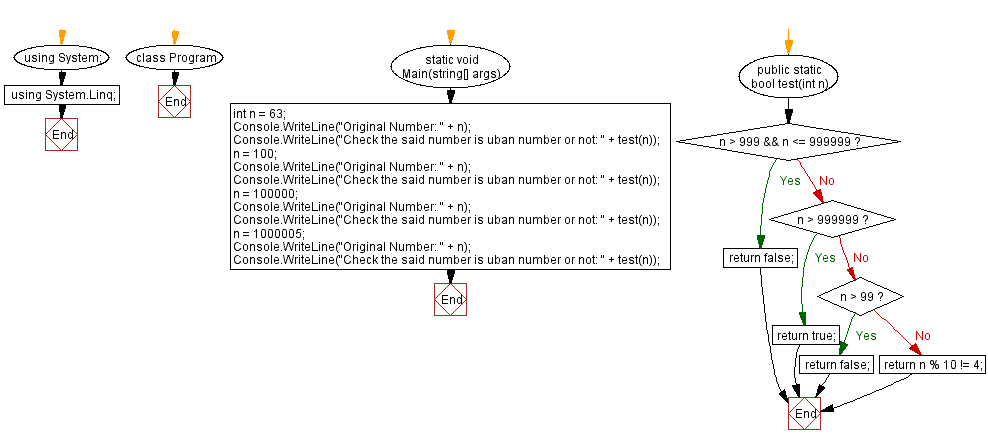
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Closest Palindrome Number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.