C#: Determine the sign of a single value and display it to the console
Write a C# Sharp program to determine the sign of a single value and display it on the console.
A number that indicates the sign of value, as shown in the following table.
Return value |
Meaning |
---|---|
-1 | value is less than zero. |
0 | value is equal to zero. |
1 | value is greater than zero. |
Sample Solution:
C# Sharp Code:
using System;
using System.Text;
namespace exercises {
class Program {
public static void Main() {
string str = "{0}: {1,3} is {2} zero.";
string nl = Environment.NewLine;
byte xByte1 = 0;
short xShort1 = -2;
int xInt1 = -3;
long xLong1 = -4;
float xSingle1 = 2.10f;
double xDouble1 = 6.0;
Decimal xDecimal1 = -7m;
Console.WriteLine($"{nl}Test the sign of the following types of values:");
Console.WriteLine(str, "Byte ", xByte1, Test(Math.Sign(xByte1)));
Console.WriteLine(str, "Int16 ", xShort1, Test(Math.Sign(xShort1)));
Console.WriteLine(str, "Int32 ", xInt1, Test(Math.Sign(xInt1)));
Console.WriteLine(str, "Int64 ", xLong1, Test(Math.Sign(xLong1)));
Console.WriteLine(str, "Single ", xSingle1, Test(Math.Sign(xSingle1)));
Console.WriteLine(str, "Double ", xDouble1, Test(Math.Sign(xDouble1)));
Console.WriteLine(str, "Decimal", xDecimal1, Test(Math.Sign(xDecimal1)));
}
public static string Test(int compare) {
if (compare == 0)
return "equal to";
else if (compare < 0)
return "less than";
else
return "greater than";
}
}
}
Sample Output:
Test the sign of the following types of values: Byte : 0 is equal to zero. Int16 : -2 is less than zero. Int32 : -3 is less than zero. Int64 : -4 is less than zero. Single : 2.1 is greater than zero. Double : 6 is greater than zero. Decimal: -7 is less than zero.
Flowchart:
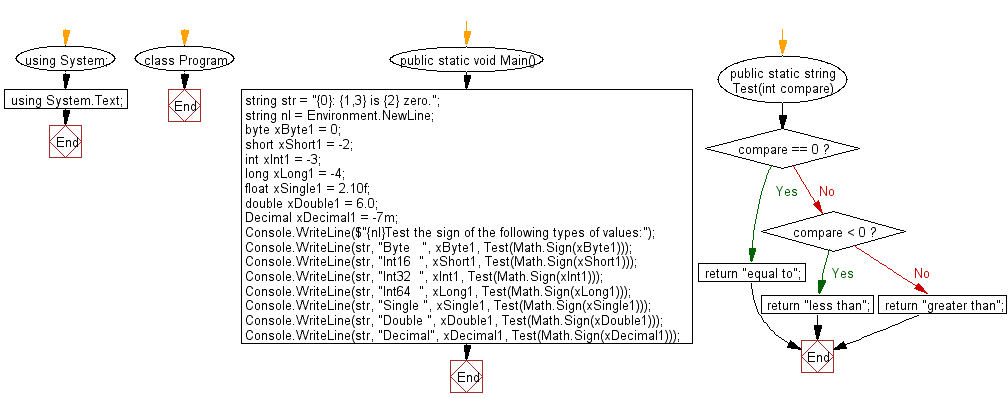
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to calculate true mean value, mean with rounding away from zero and mean with rounding to nearest of some specified decimal values.
Next: Write a C# Sharp program to calculate the of each city's size in square from the given area of some cities in the United States.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.