C#: Calculate the of each city's size in square from the given area of some cities in the United States
C# Sharp Math: Exercise-6 with Solution
The square root of the area of a square represents the length of any side of the square.
Write a C# Sharp program to calculate each city's square area based on the given area of some cities in the United States.
Sample Solution:
C# Sharp Code:
using System;
using System.Text;
namespace exercises {
class Program {
public static void Main() {
// Create an array containing the area of some squares.
Tuple < string, double > [] areas = {
Tuple.Create("Sitka, Alaska", 2870.3),
Tuple.Create("New York City", 302.6),
Tuple.Create("Los Angeles", 468.7),
Tuple.Create("Detroit", 138.8),
Tuple.Create("Chicago", 227.1),
Tuple.Create("San Diego", 325.2)
};
Console.WriteLine("{0,-18} {1,14:N1} {2,30}\n", "City", "Area (mi.)",
"Equivalent to a square with:");
foreach(var area in areas)
Console.WriteLine("{0,-18} {1,14:N1} {2,14:N2} miles per side",
area.Item1, area.Item2, Math.Round(Math.Sqrt(area.Item2), 2));
}
}
}
Sample Output:
City Area (mi.) Equivalent to a square with: Sitka, Alaska 2,870.3 53.58 miles per side New York City 302.6 17.40 miles per side Los Angeles 468.7 21.65 miles per side Detroit 138.8 11.78 miles per side Chicago 227.1 15.07 miles per side San Diego 325.2 18.03 miles per side
Flowchart:
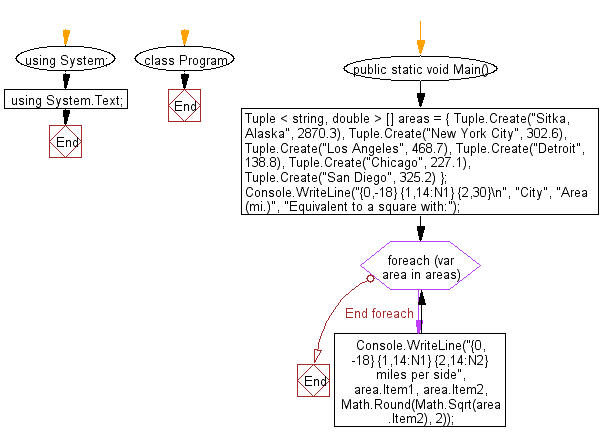
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to determine the sign of a single value and display it to the console.
Next: Write a C# Sharp program to find the whole number and fractional part from a positive and a negative Decimal number, Double number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics