C#: Insert a substring before the first occurrence of a string
C# Sharp String: Exercise-20 with Solution
Write a program in C# Sharp to insert a substring before the first occurrence of a string.
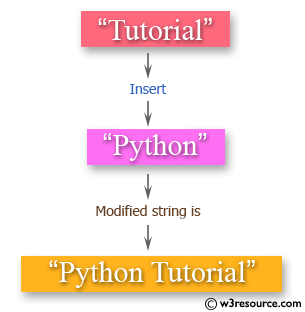
Sample Solution:-
C# Sharp Code:
using System;
// Define the Exercise20 class
public class Exercise20
{
// Main method - entry point of the program
public static void Main()
{
// Declare variables to store user input and index
string str1;
string findstring;
string insertstring;
int i;
// Prompt the user to input the original string
Console.Write("\n\nInsert a substring before the first occurrence of a string :\n");
Console.Write("--------------------------------------------------------------\n");
Console.Write("Input the original string : ");
str1 = Console.ReadLine();
// Prompt the user to input the string to be searched for
Console.Write("Input the string to be searched for : ");
findstring = Console.ReadLine();
// Prompt the user to input the string to be inserted
Console.Write("Input the string to be inserted : ");
insertstring = Console.ReadLine();
// Locate the position of the first occurrence of the string to be found
i = str1.IndexOf(findstring);
// Modify the insert string for formatting purposes
insertstring = " " + insertstring.Trim() + " ";
// Insert the insert string before the first occurrence of the found string
str1 = str1.Insert(i, insertstring);
// Display the modified string
Console.Write("The modified string is : {0}\n\n", str1);
}
}
Sample Output:
Insert a substing before the first occurence of a string : -------------------------------------------------------------- Input the original string : The string is str Input the string to be searched for : string Input the string to be inserted : original The modified string is : The original string is str
Flowchart:
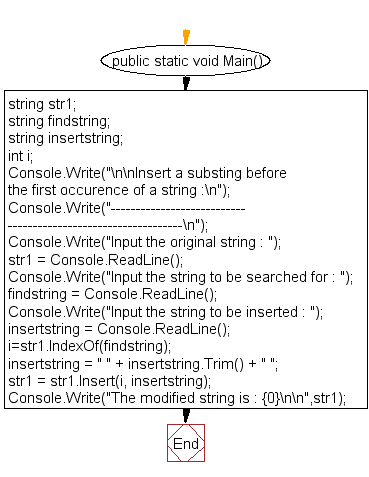
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to find the number of times a substring appears in the given string.
Next: Write a C# Sharp program to compare (less than, greater than, equal to ) two substrings.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/csharp-exercises/string/csharp-string-exercise-20.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics