C#: Demonstrate Compare method is equivalent to using ToUpper or ToLower when comparing strings
Write a C# Sharp program to demonstrate that the Compare(String, String, Boolean) method is equivalent to using ToUpper or ToLower when comparing strings.
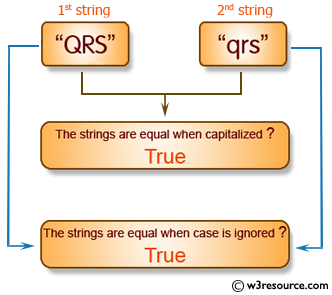
Sample Solution:-
C# Sharp Code:
using System;
class Example26
{
static void Main()
{
// Create upper-case characters from their Unicode code units.
String stringUpper = "\x0051\x0052\x0053";
// Create lower-case characters from their Unicode code units.
String stringLower = "\x0071\x0072\x0073";
// Display the strings.
Console.WriteLine("Comparing '{0}' and '{1}':",
stringUpper, stringLower);
// Compare the uppercased strings; the result is true.
// Check if the uppercased versions of both strings are equal.
Console.WriteLine("The Strings are equal when capitalized? {0}",
String.Compare(stringUpper.ToUpper(), stringLower.ToUpper()) == 0
? "true" : "false");
// The previous method call is equivalent to this Compare method, which ignores case.
// Compare the strings while ignoring the case.
Console.WriteLine("The Strings are equal when case is ignored? {0}",
String.Compare(stringUpper, stringLower, true) == 0
? "true" : "false" );
}
}
Sample Output:
Comparing 'QRS' and 'qrs': The Strings are equal when capitalized? true The Strings are equal when case is ignored? true
Flowchart :
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to compares four sets of words by using each member of the StringComparison enumeration. The comparisons use the conventions of the English (United States) and Sami (Upper Sweden) cultures.
Next: Write a C# Sharp program to demonstrate how culture can affect a comparison.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.