C#: Compare four sets of words by using each member of the StringComparison enumeration
Write a C# Sharp program to compares four sets of words by using each member of the string comparison enumeration. The comparisons use the conventions of the English (United States) and Sami (Upper Sweden) cultures.
Note: that the strings "encyclopedia" and "encyclopedia" are considered equivalent in the en-US culture but not in the Sami (Northern Sweden) culture.
Sample Solution:-
C# Sharp Code:
using System;
using System.Globalization;
using System.Threading;
public class Example25
{
public static void Main()
{
// Define an array of culture names
String[] cultureNames = { "en-AU", "sv-SE" };
// Define arrays of strings for comparison
String[] strs1 = { "case", "encyclopedia", "encyclopedia", "Archeology" };
String[] strs2 = { "Case", "encyclopedia", "encyclopedia", "ARCHEOLOGY" };
// Get all possible string comparison types
StringComparison[] comparisons = (StringComparison[])Enum.GetValues(typeof(StringComparison));
// Iterate through each culture
foreach (var cultureName in cultureNames) {
// Set the current thread's culture to the specified culture
Thread.CurrentThread.CurrentCulture = CultureInfo.CreateSpecificCulture(cultureName);
Console.WriteLine("Current Culture: {0}", CultureInfo.CurrentCulture.Name);
// Loop through each pair of strings for comparison
for (int ctr = 0; ctr <= strs1.GetUpperBound(0); ctr++) {
// Iterate through each string comparison type
foreach (var comparison in comparisons)
// Display the result of string comparison using the specified culture and comparison type
Console.WriteLine(" {0} = {1} ({2}): {3}", strs1[ctr],
strs2[ctr], comparison,
String.Equals(strs1[ctr], strs2[ctr], comparison));
Console.WriteLine(); // Add a line break after all comparisons of a pair of strings
}
Console.WriteLine(); // Add a line break after all pairs of strings for a culture
}
}
}
Sample Output:
Current Culture: en-AU case = Case (CurrentCulture): False case = Case (CurrentCultureIgnoreCase): True case = Case (InvariantCulture): False case = Case (InvariantCultureIgnoreCase): True case = Case (Ordinal): False case = Case (OrdinalIgnoreCase): True ------ Archeology = ARCHEOLOGY (CurrentCulture): False Archeology = ARCHEOLOGY (CurrentCultureIgnoreCase): True Archeology = ARCHEOLOGY (InvariantCulture): False Archeology = ARCHEOLOGY (InvariantCultureIgnoreCase): True Archeology = ARCHEOLOGY (Ordinal): False Archeology = ARCHEOLOGY (OrdinalIgnoreCase): True
Flowchart :
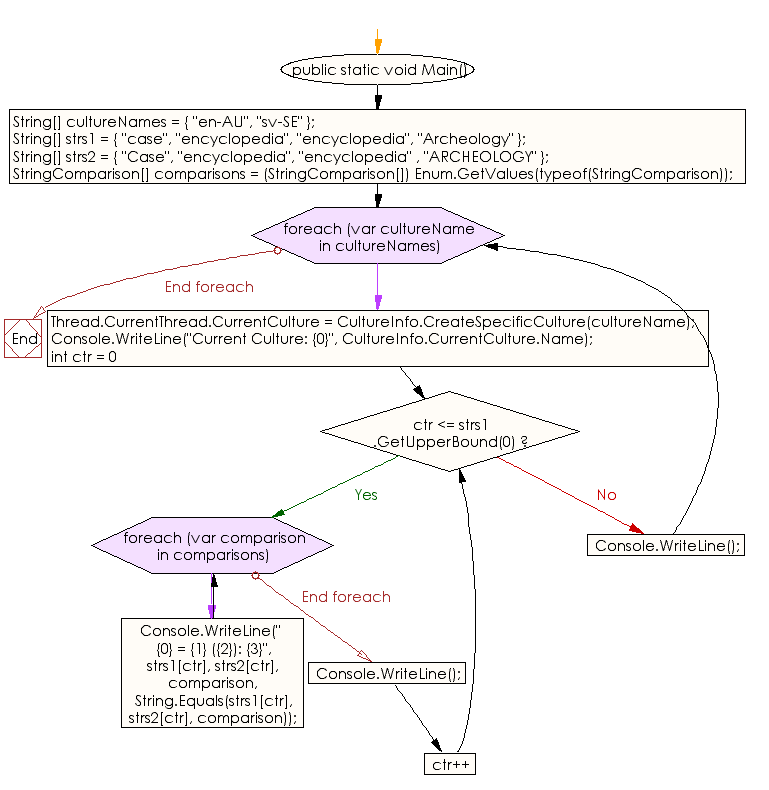
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to compare the last names of two people. It then lists them in alphabetical order.
Next: Write C# Sharp program to demonstrate that the Compare(String, String, Boolean) method is equivalent to using ToUpper or ToLower when comparing strings.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.