C#: Perform an ordinal comparison of two strings that only differ in case
Write a C# Sharp program to perform an ordinal comparison of two strings that only differ in case..
Sample Solution:-
C# Sharp Code:
using System;
class Example31
{
public static void Main()
{
// Declare two strings for comparison: "JAVA" and "python".
String str1 = "JAVA";
String str2 = "python";
String str;
int result;
// Output the strings that will be compared.
Console.WriteLine();
Console.WriteLine("Compare the numeric values of the corresponding Char objects in each string.");
Console.WriteLine("str1 = '{0}', str2 = '{1}'", str1, str2);
// Perform an ordinal comparison using String.CompareOrdinal on the strings.
result = String.CompareOrdinal(str1, str2);
// Determine the relationship between the strings based on the result of the comparison.
str = ((result < 0) ? "less than" : ((result > 0) ? "greater than" : "equal to"));
// Output the result of the comparison between the strings.
Console.Write("String '{0}' is ", str1);
Console.Write("{0} ", str);
Console.WriteLine("String '{0}'.", str2);
}
}
Sample Output:
Compare the numeric values of the corresponding Char objects in each string. str1 = 'JAVA', str2 = 'python' String 'JAVA' is less than String 'python'.
Flowchart :
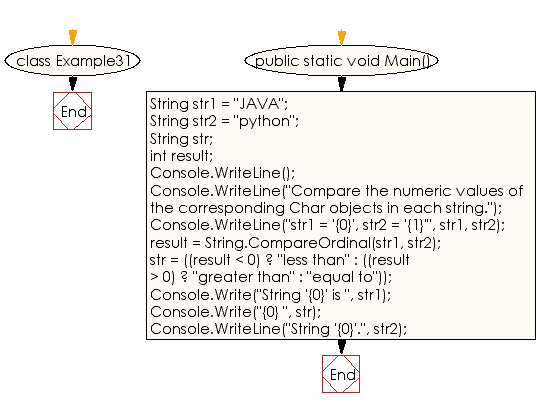
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to demonstrate that CompareOrdinal and Compare use different sort orders.
Next: Write a C# Sharp program to compare a given string with set of strings.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.