C#: Compare a given string with set of strings
Write a C# Sharp program to compare a given string with a set of strings.
Sample Solution:-
C# Sharp Code:
using System;
public class TestClass { } // Define a custom TestClass.
public class Example32
{
public static void Main()
{
// Instantiate a TestClass object.
var test = new TestClass();
// Create an array of objects for comparison.
Object[] objectsToCompare = { test, test.ToString(), 123,
123.ToString(), "some text",
"Some Text" };
string s = "some text"; // Define a string for comparison.
// Iterate through each object in the objectsToCompare array.
foreach (var objectToCompare in objectsToCompare)
{
try
{
// Attempt to compare the string 's' with the current object.
int i = s.CompareTo(objectToCompare);
Console.WriteLine("Comparing '{0}' with '{1}': {2}",
s, objectToCompare, i);
}
catch (ArgumentException)
{
// Handle the exception if the comparison is invalid.
Console.WriteLine("Bad argument: {0} (type {1})",
objectToCompare,
objectToCompare.GetType().Name);
}
}
}
}
Sample Output:
Bad argument: TestClass (type TestClass) Comparing 'some text' with 'TestClass': -1 Bad argument: 123 (type Int32) Comparing 'some text' with '123': 1 Comparing 'some text' with 'some text': 0 Comparing 'some text' with 'Some Text': -1
Flowchart :
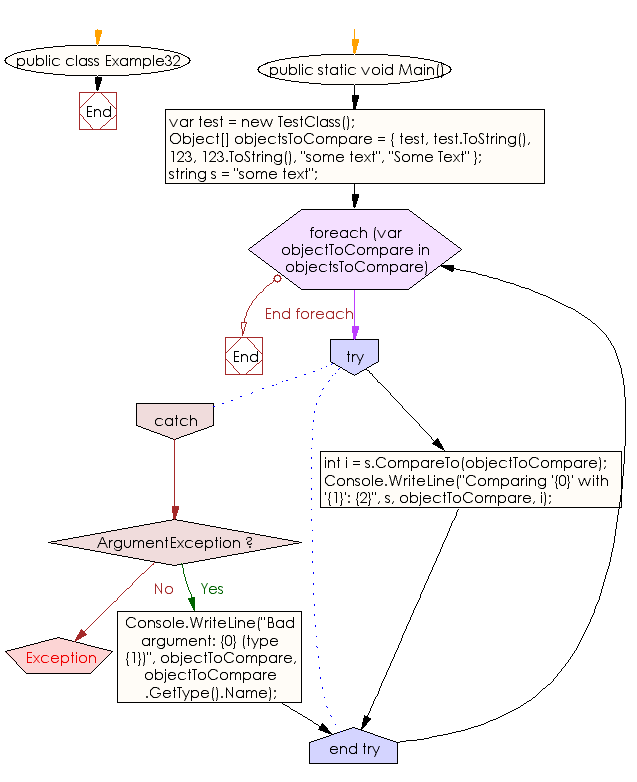
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to perform and ordinal comparison of two strings that only differ in case.
Next: Write a C# Sharp program to compare the current string instance with another string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.