C#: Compare the current string instance with another string
C# Sharp String: Exercise-33 with Solution
Write a C# Sharp program to compare the current string instance with another string.
Sample Solution:-
C# Sharp Code:
using System;
public class Example33
{
public static void Main()
{
// Define multiple strings for comparison.
string str1 = "Goodbye";
string str2 = "Hello";
string str3 = "a small string";
string str4 = "goodbye";
// Compare a string to itself.
Console.WriteLine(CompareStrings(str1, str1));
// Compare different strings and display the comparison results.
Console.WriteLine(CompareStrings(str1, str2));
Console.WriteLine(CompareStrings(str1, str3));
// Compare a string to another string that differs only by case.
Console.WriteLine(CompareStrings(str1, str4));
Console.WriteLine(CompareStrings(str4, str1));
}
// Custom method to compare two strings and return the relationship between them.
private static string CompareStrings(string str1, string str2)
{
// Compare the values using the CompareTo method on the first string.
int cmpVal = str1.CompareTo(str2);
// Determine the relationship between the strings based on the comparison result.
if (cmpVal == 0) // The strings are identical.
return "The strings occur in the same position in the sort order.";
else if (cmpVal > 0) // The first string precedes the second in the sort order.
return "The first string precedes the second in the sort order.";
else // The first string follows the second in the sort order.
return "The first string follows the second in the sort order.";
}
}
Sample Output:
The strings occur in the same position in the sort order. The first string follows the second in the sort order. The first string precedes the second in the sort order. The first string precedes the second in the sort order. The first string follows the second in the sort order.
Flowchart :
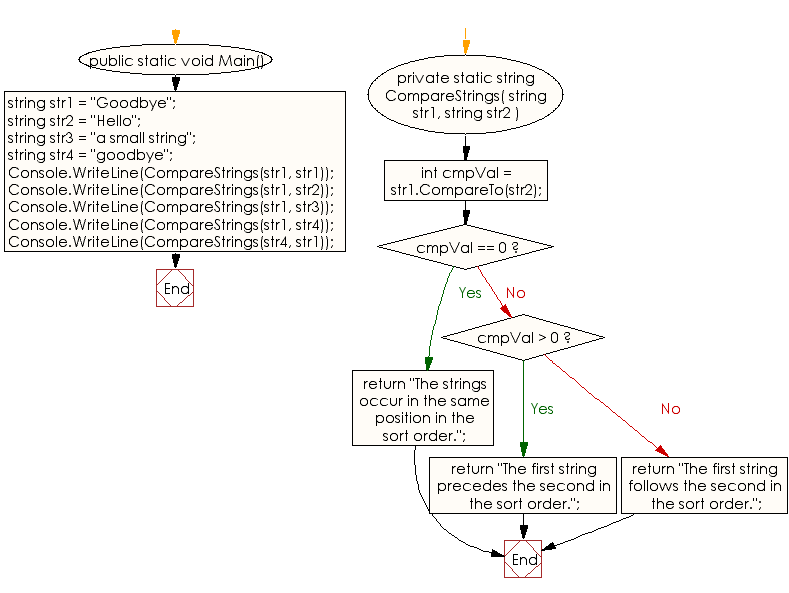
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to compare a given string with set of strings.
Next: Write a C# Sharp program to concatenate three objects, objects with a variable and 3-element object array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/csharp-exercises/string/csharp-string-exercise-33.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics