C#: Concatenate three objects, objects with a variable and 3-element object array
Write a C# Sharp program to concatenate three objects, objects with a variable and a 3-element object array.
Sample Solution:-
C# Sharp Code:
using System;
class Example34
{
public static void Main()
{
int i = -123;
Object o = i; // Assigning an integer value to an object reference.
Object[] objs = new Object[] { -123, -456, -789 }; // Creating an object array.
// Concatenate different combinations of objects and display the results.
Console.WriteLine("Concatenate 1, 2, and 3 objects:");
Console.WriteLine("1) {0}", String.Concat(o)); // Concatenating a single object.
Console.WriteLine("2) {0}", String.Concat(o, o)); // Concatenating two objects.
Console.WriteLine("3) {0}", String.Concat(o, o, o)); // Concatenating three objects.
Console.WriteLine("\nConcatenate 4 objects and a variable length parameter list:");
Console.WriteLine("4) {0}", String.Concat(o, o, o, o)); // Concatenating four objects.
Console.WriteLine("5) {0}", String.Concat(o, o, o, o, o)); // Concatenating five objects.
Console.WriteLine("\nConcatenate a 3-element object array:");
Console.WriteLine("6) {0}", String.Concat(objs)); // Concatenating objects from an array.
}
}
Sample Output:
Concatenate 1, 2, and 3 objects: 1) -123 2) -123-123 3) -123-123-123 Concatenate 4 objects and a variable length parameter list: 4) -123-123-123-123 5) -123-123-123-123-123 Concatenate a 3-element object array: 6) -123-456-789
Flowchart :
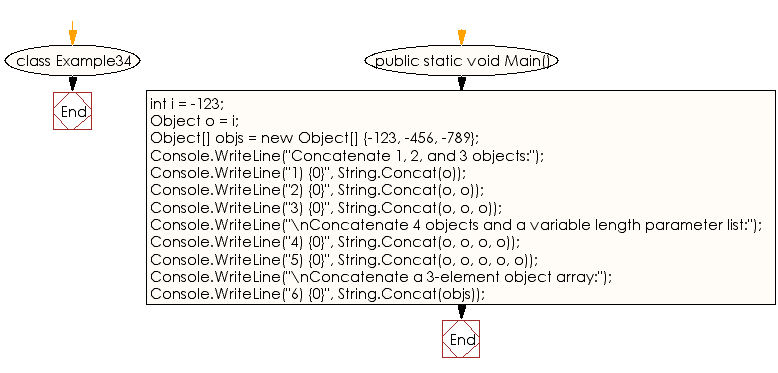
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to compare the current string instance with another string.
Next: Write a C# Sharp program to concatenate a list of variable parameters.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.