C#: Concatenate a list of variable parameters
Write a C# Sharp program to concatenate a list of variable parameters.
Sample Solution:-
C# Sharp Code:
using System;
using System.Collections;
public class Example35
{
public static void Main()
{
const int WORD_SIZE = 4; // Constant representing the word size.
// Define some 4-letter words to be scrambled.
string[] words = { "abcd", "efgh", "ijkl", "mnop" };
// Define two arrays equal to the number of letters in each word.
double[] keys = new double[WORD_SIZE]; // Array to hold random numbers as keys.
string[] letters = new string[WORD_SIZE]; // Array to hold individual letters.
// Initialize the random number generator.
Random rnd = new Random();
// Scramble each word.
foreach (string word in words)
{
for (int ctr = 0; ctr < word.Length; ctr++)
{
// Populate the array of keys with random numbers.
keys[ctr] = rnd.NextDouble();
// Assign a letter to the array of letters.
letters[ctr] = word[ctr].ToString();
}
// Sort the array.
Array.Sort(keys, letters, 0, WORD_SIZE, Comparer.Default);
// Display the scrambled word.
string scrambledWord = String.Concat(letters[0], letters[1],
letters[2], letters[3]);
Console.WriteLine("{0} --> {1}", word, scrambledWord);
}
}
}
Sample Output:
abcd --> cadb efgh --> efhg ijkl --> iklj mnop --> nmop
Flowchart :
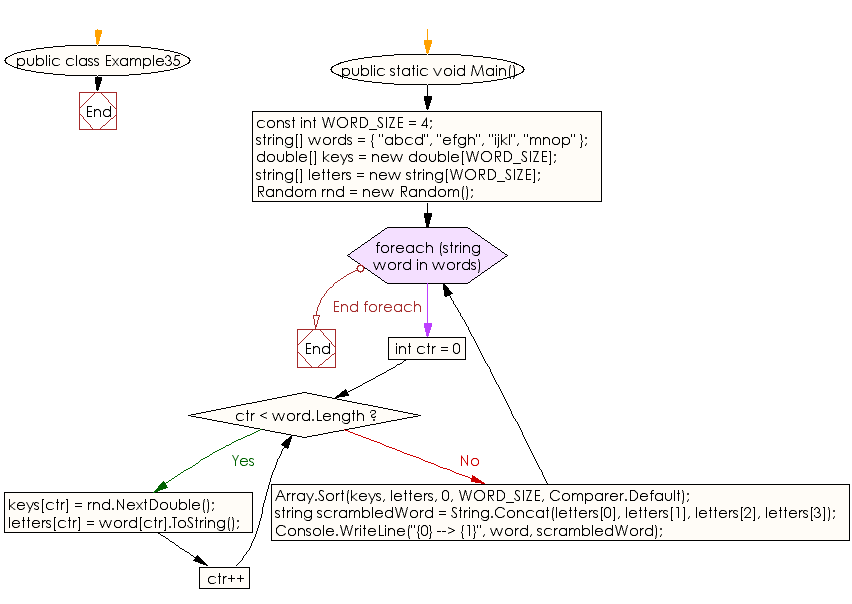
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to concatenate three objects, objects with a variable and 3-element object array.
Next: Write a C# Sharp program to concatenate three strings and display the result.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.