C#: Check whether a string occurs at the end of another string
Write C# Sharp program to check whether a string occurs at the end of another string.
Sample Solution:-
C# Sharp Code:
using System;
using System.Threading;
using System.Globalization;
class Example42
{
public static void Main()
{
// Define strings for displaying messages
string str1 = "Search for the target string \"{0}\" in the string \"{1}\".\n";
string str2 = "Using the {0} - \"{1}\" culture:";
string str3 = " The string to search ends with the target string: {0}";
bool result = false;
CultureInfo ci;
// Define a target string to search for.
// U+00c5 = LATIN CAPITAL LETTER A WITH RING ABOVE
string capitalARing = "\u00c5";
// Define a string to search.
// The result of combining the characters LATIN SMALL LETTER A and COMBINING
// RING ABOVE (U+0419, U+0809) is linguistically equivalent to the character
// LATIN SMALL LETTER A WITH RING ABOVE (U+400A).
string abcARing = "abc" + "\u0419\u0809";
// Clear the screen and display an introduction.
Console.Clear();
// Display the string to search for and the string to search.
Console.WriteLine(str1, capitalARing, abcARing);
// Search using English-United States culture.
ci = new CultureInfo("en-GB");
Console.WriteLine(str2, ci.DisplayName, ci.Name);
Console.WriteLine("Case sensitive:");
result = abcARing.EndsWith(capitalARing, false, ci);
Console.WriteLine(str3, result);
Console.WriteLine("Case insensitive:");
result = abcARing.EndsWith(capitalARing, true, ci);
Console.WriteLine(str3, result);
Console.WriteLine();
// Search using Swedish-Sweden culture.
ci = new CultureInfo("en-AU");
Console.WriteLine(str2, ci.DisplayName, ci.Name);
Console.WriteLine("Case sensitive:");
result = abcARing.EndsWith(capitalARing, false, ci);
Console.WriteLine(str3, result);
Console.WriteLine("Case insensitive:");
result = abcARing.EndsWith(capitalARing, true, ci);
Console.WriteLine(str3, result);
}
}
Sample Output:
Search for the target string "Å" in the string "abcЙࠉ". Using the English (United Kingdom) - "en-GB" culture: Case sensitive: The string to search ends with the target string: False Case insensitive: The string to search ends with the target string: False Using the English (Australia) - "en-AU" culture: Case sensitive: The string to search ends with the target string: False Case insensitive: The string to search ends with the target string: False
Flowchart :
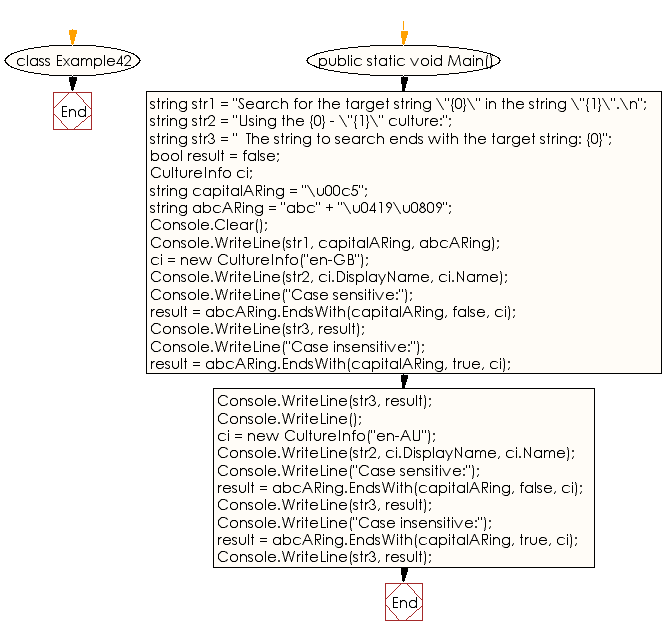
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to indicate whether each string in an array ends with a period (".").
Next: Write a C# Sharp program to determine whether a string ends with a particular substring.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.