C#: Determine whether a string ends with a particular substring
Write a C# Sharp program to determine whether a string ends with a particular substring.
The results are affected by the choice of culture, whether case is ignored, and whether an ordinal comparison is performed.
Sample Solution:-
C# Sharp Code:
using System;
using System.Threading;
class Example43
{
public static void Main()
{
// Define the input string for the demonstration
string str = "Determine whether a string ends with another string, " +
"using\n different values of StringComparison.";
// Define the StringComparison values to test
StringComparison[] scValues = {
StringComparison.CurrentCulture,
StringComparison.CurrentCultureIgnoreCase,
StringComparison.InvariantCulture,
StringComparison.InvariantCultureIgnoreCase,
StringComparison.Ordinal,
StringComparison.OrdinalIgnoreCase };
// Clear the console and display the initial string
Console.Clear();
Console.WriteLine(str);
// Display the current culture to emphasize its effect on comparison
Console.WriteLine("The current culture is {0}.\n",
Thread.CurrentThread.CurrentCulture.Name);
// Determine whether different string comparison methods result in strings ending
foreach (StringComparison sc in scValues)
{
Console.WriteLine("StringComparison.{0}:", sc);
Test("xyzPQR", "PQR", sc); // Test if the first string ends with the second string
Test("xyzPQR", "PQR", sc); // Test if the first string ends with the second string
Console.WriteLine();
}
}
// Method to perform the string comparison test
protected static void Test(string x, string y, StringComparison comparison)
{
string resultFmt = "\"{0}\" {1} with \"{2}\".";
string result = "does not end";
// Check if the first string ends with the second string using the specified comparison
if (x.EndsWith(y, comparison))
result = "ends";
// Display the result of the comparison
Console.WriteLine(resultFmt, x, result, y);
}
}
Sample Output:
StringComparison.CurrentCultureIgnoreCase: "xyzPQR" ends with "PQR". "xyzPQR" ends with "PQR". StringComparison.InvariantCulture: "xyzPQR" ends with "PQR". "xyzPQR" ends with "PQR". StringComparison.InvariantCultureIgnoreCase: "xyzPQR" ends with "PQR". "xyzPQR" ends with "PQR". StringComparison.Ordinal: "xyzPQR" ends with "PQR". "xyzPQR" ends with "PQR". StringComparison.OrdinalIgnoreCase: "xyzPQR" ends with "PQR". "xyzPQR" ends with "PQR".
Flowchart :
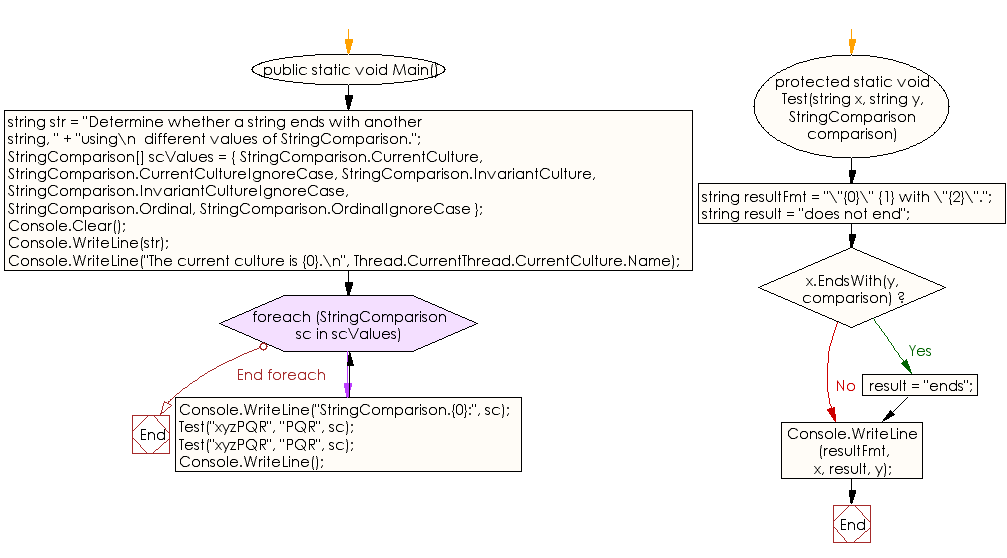
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write C# Sharp program to check whether a string occurs at the end of another string.
Next: Write a C# Sharp program to get the longest Palindromic substring from a given string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.