C#: Remove duplicate characters from a given string
Write a C# Sharp program to remove duplicate characters from a given string.
Sample Solution:-
C# Sharp Code:
using System;
using System.Collections.Generic;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
// Define and display original string
String str1;
str1 = "aaaaaabbbbccc";
Console.WriteLine("Original String: " + str1);
// Display the string after removing duplicate characters
Console.WriteLine("After removing duplicates characters from the said string:");
Console.WriteLine(remove_duplicate_chars(str1));
// Repeat the process for different strings
str1 = "Python";
Console.WriteLine("Original String: " + str1);
Console.WriteLine("After removing duplicates characters from the said string:");
Console.WriteLine(remove_duplicate_chars(str1));
str1 = "Java";
Console.WriteLine("Original String: " + str1);
Console.WriteLine("After removing duplicates characters from the said string:");
Console.WriteLine(remove_duplicate_chars(str1));
}
// Method to remove duplicate characters from a string
public static string remove_duplicate_chars(string str1)
{
var indexes = new Dictionary<char, int>();
// Store the last index of each character
for (int i = 0; i < str1.Length; i++)
indexes[str1[i]] = i;
var flag = new HashSet<char>();
var stack = new Stack<char>();
for (int i = 0; i < str1.Length; i++)
{
var ele = str1[i];
if (!flag.Contains(ele))
{
// Remove elements from the stack that are greater than current and occur later in the string
while (stack.Count > 0 && stack.Peek() > ele && i < indexes[stack.Peek()])
flag.Remove(stack.Pop());
flag.Add(ele);
stack.Push(ele);
}
}
var s = new char[stack.Count];
int index = stack.Count - 1;
// Reverse the stack content to restore original order
foreach (var ele in stack)
s[index--] = ele;
return new string(s);
}
}
}
Sample Output:
Original String: aaaaaabbbbccc After removing duplicates characters from the said string: abc Original String: Python After removing duplicates characters from the said string: Python Original String: Java After removing duplicates characters from the said string: Jav
Flowchart :
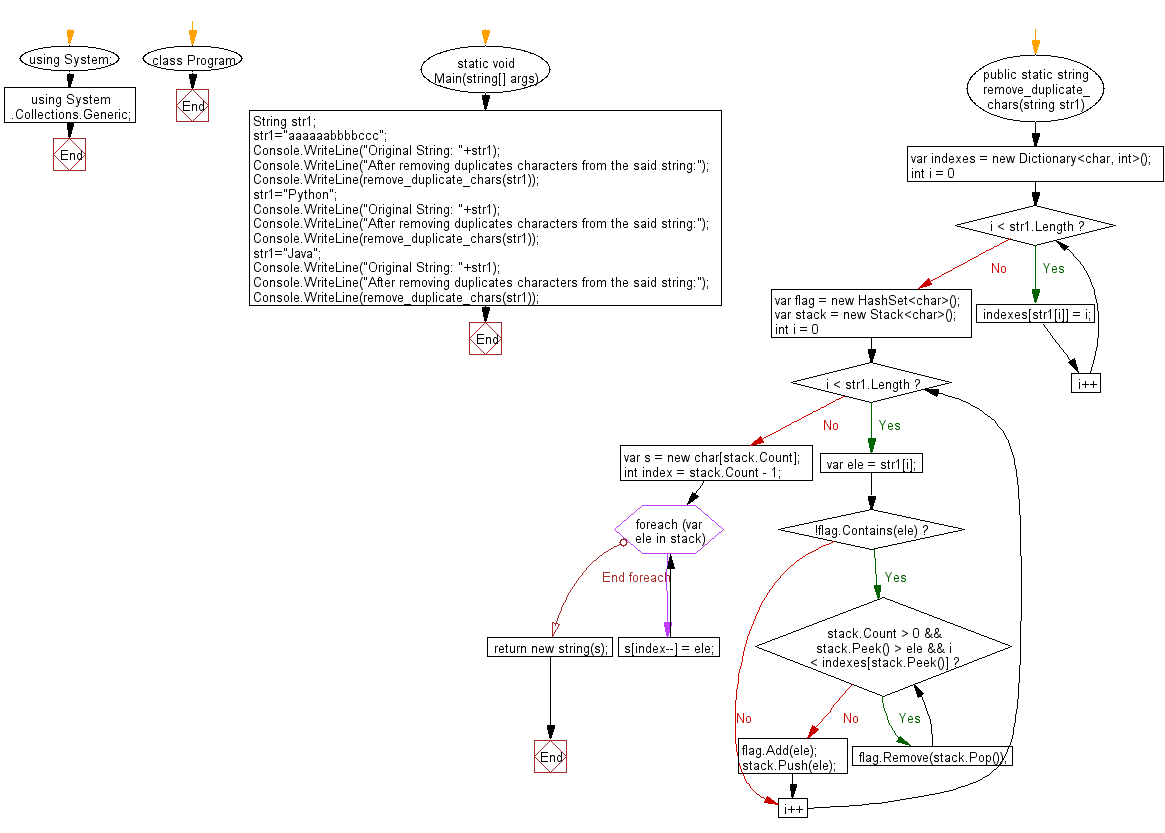
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to reverse a given string in uppercase.
Next: Write a C# Sharp program to find the length of the longest substring without repeating characters from a given string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.