C#: Find the length of the longest substring without repeating characters from a given string
Write a C# Sharp program to find the length of the longest substring without repeating characters in a given string.
Note:
1) Given string consists of English letters, digits, symbols and spaces.
2) 0 <= Given string length <= 5 * 104
Difficulty: Medium.
Company: Amazon, Google, Bloomberg, Microsoft, Adobe, Apple, Oracle, Facebook and more.
Input String : pickoutthelongestsubstring
The longest substring : ubstring
The longest Substring Length : 8
Input String : ppppppppppppp
The longest substring : p
The longest Substring Length : 1
Input String : Microsoft
The longest substring : Micros
The longest Substring Length : 6
Sample Solution:
C# Sharp Code:
using System;
using System.Collections.Generic;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
// Define and display original string
String str1;
str1 = "aaaaaabbbbccc";
Console.WriteLine("Original String: " + str1);
// Calculate and display the length of the longest substring without repeating characters
Console.WriteLine("Length of the longest substring without repeating characters of the said string:");
Console.WriteLine(without_repeated_chars_longest_substring(str1));
// Repeat the process for different strings
str1 = "BDEFGAABEF";
Console.WriteLine("Original String: " + str1);
Console.WriteLine("Length of the longest substring without repeating characters of the said string:");
Console.WriteLine(without_repeated_chars_longest_substring(str1));
str1 = "Python";
Console.WriteLine("Original String: " + str1);
Console.WriteLine("Length of the longest substring without repeating characters of the said string:");
Console.WriteLine(without_repeated_chars_longest_substring(str1));
str1 = "Java";
Console.WriteLine("Original String: " + str1);
Console.WriteLine("Length of the longest substring without repeating characters of the said string:");
Console.WriteLine(without_repeated_chars_longest_substring(str1));
}
// Method to find the length of the longest substring without repeating characters
public static int without_repeated_chars_longest_substring(string str1)
{
if (string.IsNullOrEmpty(str1)) return 0;
// Dictionary to store character positions
var map_str = new Dictionary<char, int>();
var max_len = 0;
var last_repeat_pos = -1;
// Loop through the string
for (int i = 0; i < str1.Length; i++)
{
// Check if the character exists in the dictionary and update its last position
if (map_str.ContainsKey(str1[i]) && last_repeat_pos < map_str[str1[i]])
last_repeat_pos = map_str[str1[i]];
// Check for the maximum length of the non-repeating substring
if (max_len < i - last_repeat_pos)
max_len = i - last_repeat_pos;
// Store current character and its position in the dictionary
map_str[str1[i]] = i;
}
return max_len;
}
}
}
;
Sample Output:
Original String: aaaaaabbbbccc Length of the longest substring without repeating characters of the said string: 2 Original String: BDEFGAABEF Length of the longest substring without repeating characters of the said string: 6 Original String: Python Length of the longest substring without repeating characters of the said string: 6 Original String: Java Length of the longest substring without repeating characters of the said string: 3
Flowchart :
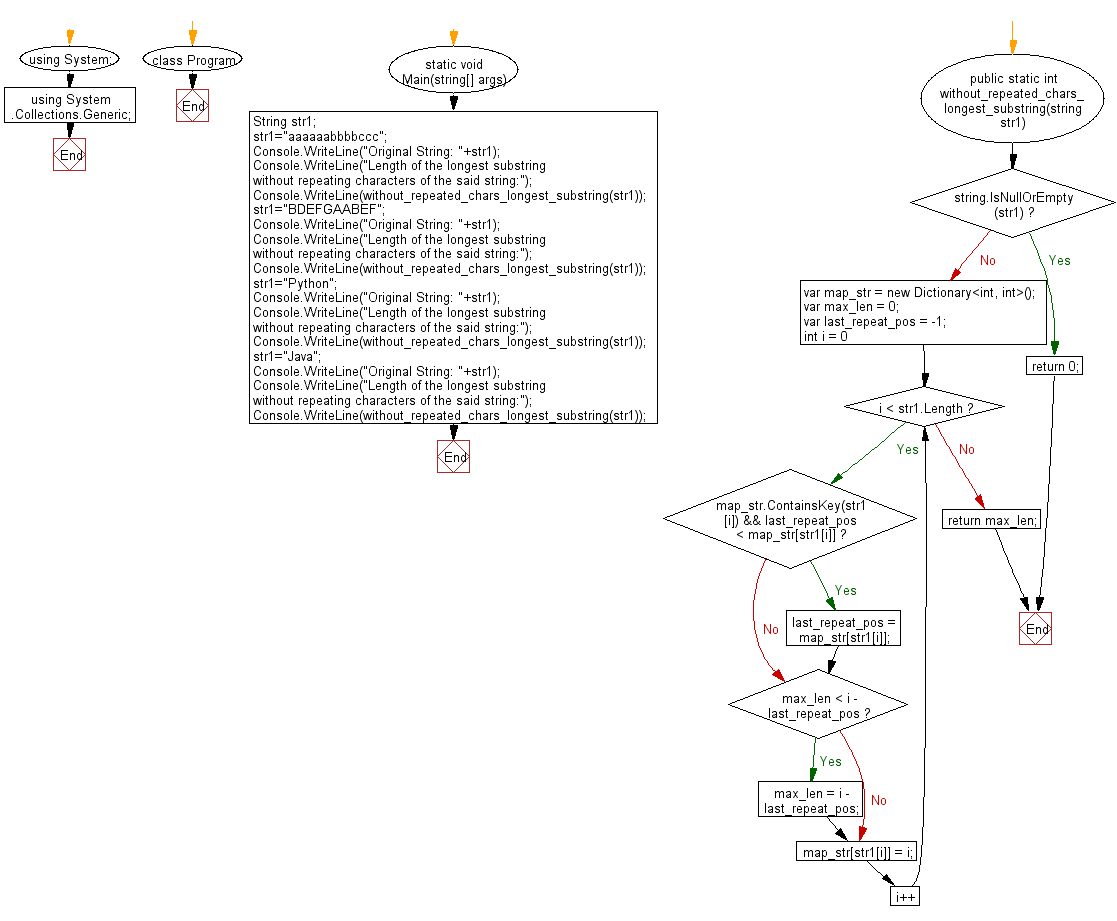
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to remove duplicate characters from a given string.
Next: Write a C# Sharp program to reverse the case (upper->lower, lower->upper) of all the characters of given string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.