C#: Reverse the case of all characters of given string
Write a C# Sharp program to reverse the case (upper->lower, lower->upper) of all the characters in a given string.
Sample Solution:-
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
// Display the original string and its case-reversed version for different inputs
Console.WriteLine("Original string: PHP");
Console.WriteLine("After reversing the case of all characters of the said string: " + test("PHP"));
Console.WriteLine("\nOriginal string: JavaScript");
Console.WriteLine("After reversing the case of all characters of the said string: " + test("JavaScript"));
Console.WriteLine("\nOriginal string: Python 3.0");
Console.WriteLine("After reversing the case of all characters of the said string: " + test("Python 3.0"));
}
// Method to reverse the case of all characters in the input string
public static string test(string text)
{
// Using LINQ, iterate through each character in the string and reverse its case
// If the character is uppercase, convert it to lowercase, and vice versa
return string.Concat(text.Select(x => char.IsUpper(x) ? char.ToLower(x) : char.ToUpper(x)));
}
}
}
Sample Output:
Original string: PHP After reversing the case of all characters of the said string: php Original string: JavaScript After reversing the case of all characters of the said string: jAVAsCRIPT Original string: Python 3.0 After reversing the case of all characters of the said string: pYTHON 3.0
Flowchart :
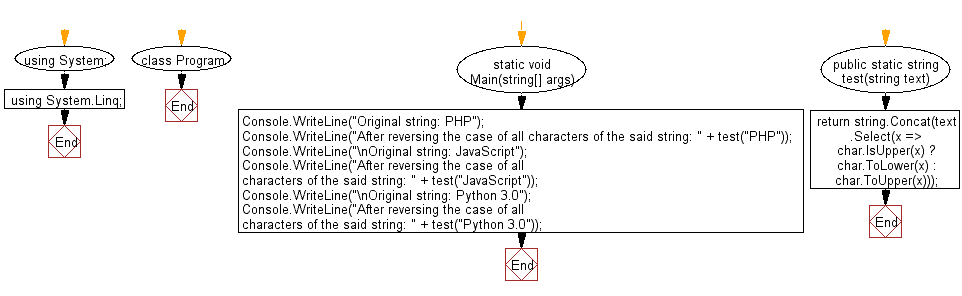
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to find the length of the longest substring without repeating characters from a given string.
Next: Write a C# Sharp program to find the middle character(s) of a given string. Return the middle character if the length of the string is odd and return two middle characters if the length of the string is even.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.