C#: Find the middle character(s) of a given string
Write a C# Sharp program to find the central character(s) in a given string. Return the middle character if the string length is odd and return two middle characters if the string length is even.
Sample Solution:-
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
// Define different input strings and display their middle character(s)
string text = "Python";
Console.WriteLine("Original string: " + text);
Console.WriteLine("Middle character(s) of the said string: " + test(text));
text = "PHP";
Console.WriteLine("\nOriginal string: " + text);
Console.WriteLine("Middle character(s) of the said string: " + test(text));
text = "C#";
Console.WriteLine("\nOriginal string: " + text);
Console.WriteLine("Middle character(s) of the said string: " + test(text));
}
// Method to retrieve the middle character(s) of the input string
public static string test(string text)
{
// Calculate the length to extract the middle character(s)
int l = 1 - text.Length % 2;
// Using Substring to get the middle character(s) of the string
// If the string length is odd, returns a single character at the middle position
// If the string length is even, returns the two characters at the middle positions
return text.Substring(text.Length / 2 - l, 1 + l);
}
}
}
Sample Output:
Original string: Python Middle character(s) of the said string: th Original string: PHP Middle character(s) of the said string: H Original string: C# Middle character(s) of the said string: C#
Flowchart :
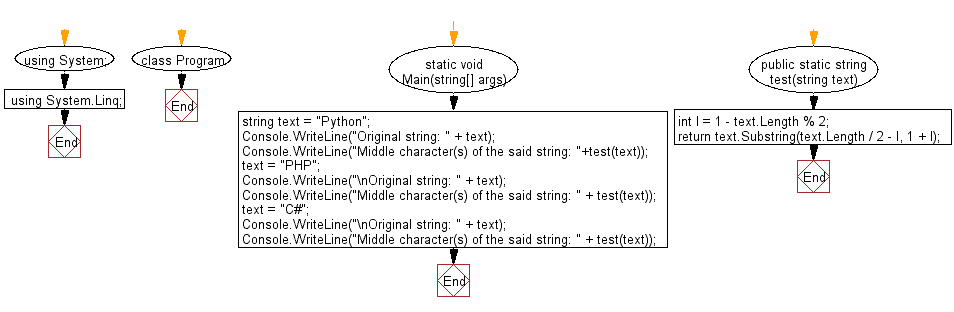
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to reverse the case (upper->lower, lower->upper) of all the characters of given string.
Next: Write a C# Sharp program to find the maximum and minimum number from a given string of numbers separated by single space.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.