C#: Maximum and minimum number from a given string of numbers separated by single space
C# Sharp String: Exercise-50 with Solution
Write a C# Sharp program to find the maximum and minimum number from a given string of numbers separated by a single space.
Sample string : "3 4 8 9 0 2 1"
Sample Solution:-
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
// Define a string containing numbers separated by spaces
string str_num = "3 4 8 9 0 2 1";
Console.WriteLine("Original string of numbers: " + str_num);
// Display the maximum and minimum numbers in the string
Console.WriteLine("Maximum and minimum number of the said string: " + test(str_num));
// Update the string with new numbers
str_num = "-2 -1 0 4 10";
Console.WriteLine("\nOriginal string of numbers: " + str_num);
// Display the maximum and minimum numbers in the updated string
Console.WriteLine("Maximum and minimum number of the said string: " + test(str_num));
}
// Method to find the maximum and minimum numbers from a string of space-separated numbers
public static string test(string str_num)
{
// Split the input string into an array of strings containing numbers,
// then convert these strings into an array of integers using Select and int.Parse
var result = str_num.Split(' ').Select(int.Parse).ToArray();
// Use the Max and Min methods on the resulting array to find the maximum and minimum numbers
return result.Max() + ", " + result.Min();
}
}
}
Sample Output:
Original string of numbers: 3 4 8 9 0 2 1 Maximum and minimum number of the said string: 9, 0 Original string of numbers: -2 -1 0 4 10 Maximum and minimum number of the said string: 10, -2
Flowchart :
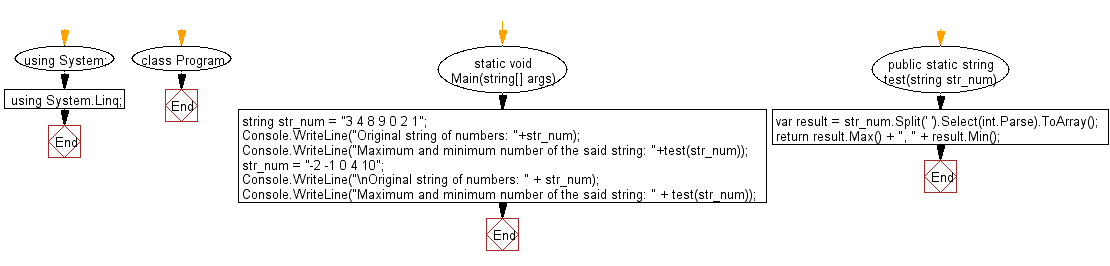
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to find the middle character(s) of a given string. Return the middle character if the length of the string is odd and return two middle characters if the length of the string is even.
Next: Write a C# Sharp program to check whether a given string is an “isograms” or not. Return True or False.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics