C#: Check a given string is an isograms or not
Write a C# Sharp program to check whether a given string is an "isograms" or not. Return True or False.
From Wikipedia,
A heterogram (from hetero-, meaning 'different', + -gram, meaning 'written') is a word, phrase, or sentence in which no letter of the alphabet occurs more than once. The terms isogram and nonpattern word have also been used to mean the same thing.
Sample Solution:-
C# Sharp Code:
using System;
using System.Linq;
using System.Collections.Generic;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
// Declare and initialize strings
string str1 = "Python";
Console.WriteLine("Original string: " + str1);
// Check if the string is an isogram and display the result
Console.WriteLine("Check the said string is an 'isograms' or not! " + test(str1));
str1 = "JavaScript";
Console.WriteLine("\nOriginal string: " + str1);
Console.WriteLine("Check the said string is an 'isograms' or not! " + test(str1));
str1 = "PHP";
Console.WriteLine("\nOriginal string: " + str1);
Console.WriteLine("Check the said string is an 'isograms' or not! " + test(str1));
str1 = "C#";
Console.WriteLine("\nOriginal string: " + str1);
Console.WriteLine("Check the said string is an 'isograms' or not! " + test(str1));
}
// Method to check if a string is an isogram
public static bool test(string str1)
{
// Get the length of the string
int str_len = str1.Length;
// Convert the string to lowercase, find distinct characters, and count them
// Check if the count of distinct characters is equal to the length of the string
return str1.ToLower().Distinct().Count() == str_len;
}
}
}
Sample Output:
Original string: Python Check the said string is an 'isograms' or not! True Original string: JavaScript Check the said string is an 'isograms' or not! False Original string: PHP Check the said string is an 'isograms' or not! False Original string: C# Check the said string is an 'isograms' or not! True
Flowchart :
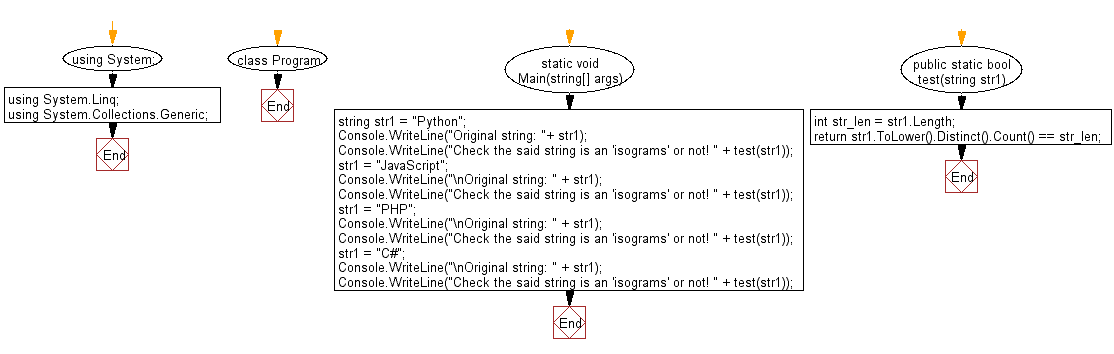
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to find the maximum and minimum number from a given string of numbers separated by single space.
Next: Write a C# Sharp program to convert the first character of each word of a given string to uppercase.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.