C#: Convert the first character of each word of a given string to uppercase
Write a C# Sharp program to convert the first character of each word in a given string to uppercase.
Letter case is the distinction between the letters that are in larger uppercase or capitals and smaller lowercase in the written representation of certain languages.
Sample Solution:-
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
// Declare and initialize a string variable
string str1 = "python exercises";
// Display the original string
Console.WriteLine("Original string: " + str1);
// Display the string after converting the first character of each word
Console.WriteLine("After converting the first character of each word of the said string:\n" + Test(str1));
// Change the value of str1
str1 = "The quick brown Fox jumps over the little lazy Dog";
// Display the new original string
Console.WriteLine("\nOriginal string: " + str1);
// Display the string after converting the first character of each word
Console.WriteLine("After converting the first character of each word of the said string:\n" + Test(str1));
}
// Define a method 'Test' that takes a string argument and returns a modified string
public static string Test(string inputString)
{
// Split the input string into words, capitalize the first character of each word, and join them back into a string
return string.Join(" ", inputString.Split(' ').Select(word => char.ToUpper(word[0]) + word.Substring(1)));
}
}
}
Sample Output:
Original string: python exercises After converting the first character of each word of the said string: Python Exercises Original string: The quick brown Fox jumps over the little lazy Dog After converting the first character of each word of the said string: The Quick Brown Fox Jumps Over The Little Lazy Dog
Flowchart :
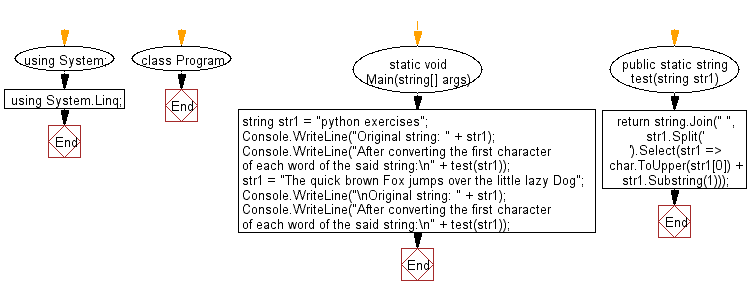
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous:Write a C# Sharp program to check whether a given string is an "isograms" or not. Return True or False.
Next: Write a C# Sharp program to find the position of a specified word in a given string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.