C#: Alternate the case of each letter in a given string and the first letter of the said string must be uppercase
Write a C# Sharp program to alternate the case of each letter in a given string. The first letter of this string must be uppercase.
Sample Solution:-
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
// Declare and initialize a string variable
string str1 = "c# Exercises";
// Display the original string
Console.WriteLine("Original string: " + str1);
// Display the string after alternating the case of each letter
Console.WriteLine("\nAfter alternating the case of each letter of the said string:\n" + test(str1));
// Change the value of str1
str1 = "C# is used to develop web apps, desktop apps, mobile apps, games and much more.";
// Display the new original string
Console.WriteLine("\nOriginal string: " + str1);
// Display the string after alternating the case of each letter
Console.WriteLine("\nAfter alternating the case of each letter of the said string:\n" + test(str1));
}
// Define a method 'test' that takes a string argument 'str' and returns a modified string with alternating case letters
public static string test(string str)
{
// Double the spaces in the text
string text = str.Replace(" ", " ");
string result_str = "";
// Capitalize the first character of the string
result_str += Char.ToUpper(str[0]);
// Loop through each character in the text, starting from the second character
for (int i = 1; i < text.Length; i++)
{
// Check if the index is even (alternating position)
if (i % 2 == 0)
result_str += Char.ToUpper(text[i]); // Convert to uppercase and add to the result string
else if (i % 2 != 0 && Char.IsUpper(text[i]))
result_str += Char.ToLower(text[i]); // Convert to lowercase and add to the result string if the character is already uppercase
else
result_str += text[i]; // Add the character as it is to the result string
}
// Replace double spaces with single spaces in the result string
result_str = result_str.Replace(" ", " ");
// Return the modified string with alternating case letters
return result_str;
}
}
}
Sample Output:
Original string: c# Exercises After alternating the case of each letter of the said string: C# ExErCiSeS Original string: C# is used to develop web apps, desktop apps, mobile apps, games and much more. After alternating the case of each letter of the said string: C# Is UsEd To DeVeLoP wEb ApPs, dEsKtOp ApPs, mObIlE aPpS, GaMeS aNd MuCh MoRe.
Flowchart :
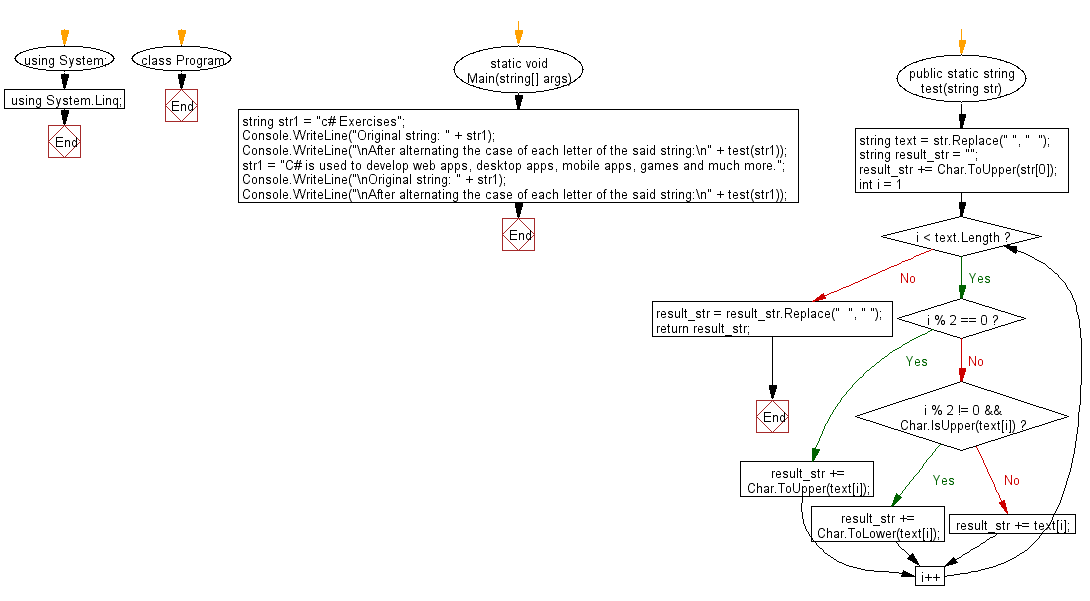
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to find the position of a specified word in a given string.
Next: Write a C# Sharp program reverse all the words of a given string which have even length.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.