C#: Reverse all the words of a given string which have even length
Write a C# Sharp program to reverse all the words of a given string with even length.
Sample Solution:-
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
// Declare and initialize a string variable
string str1 = "C# Exercises";
// Display the original string
Console.WriteLine("Original string: " + str1);
// Display the string after reversing words with even length
Console.WriteLine("\nReverse all the words of the said string which have even length.:\n" + test(str1));
// Change the value of str1
str1 = "C# is used to develop web apps , desktop apps , mobile apps , games and much more.";
// Display the new original string
Console.WriteLine("\nOriginal string: " + str1);
// Display the string after reversing words with even length
Console.WriteLine("\nReverse all the words of the said string which have even length.:\n" + test(str1));
}
// Define a method 'test' that takes a string argument 'text' and returns a modified string
public static string test(string text)
{
// Split the input text into words and check if the length of each word is even.
// If the length is even, reverse the characters; otherwise, keep the word as it is.
return string.Join(" ", text.Split(' ').Select(str => str.Length % 2 != 0 ? str : new String(str.Reverse().ToArray())));
}
}
}
Sample Output:
Original string: C# Exercises Reverse all the words of the said string which have even length.: #C Exercises Original string: C# is used to develop web apps , desktop apps , mobile apps , games and much more. Reverse all the words of the said string which have even length.: #C si desu ot develop web sppa , desktop sppa , elibom sppa , games and hcum more.
Flowchart :
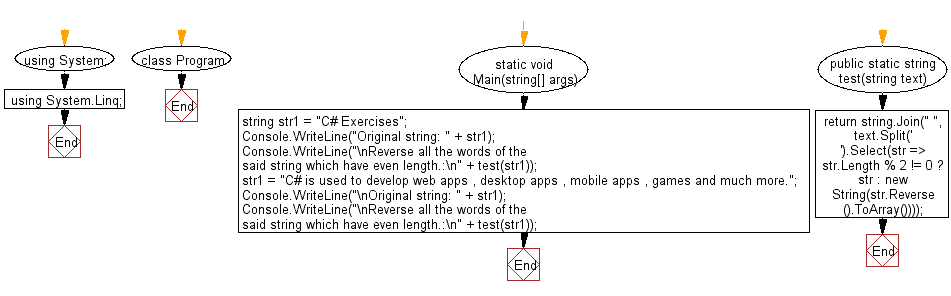
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to alternate the case of each letter in a given string and the first letter of the said string must be uppercase.
Next: Write a C# Sharp program to find the longest common ending between two given strings.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.