C#: Find the longest common ending between two given strings
C# Sharp String: Exercise-56 with Solution
Write a C# Sharp program to find the longest common ending between two given strings.
Sample Solution:
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
// Declare and initialize two string variables
string str1 = "running";
string str2 = "ruminating";
// Display the original strings
Console.WriteLine("Original strings: " + str1 + " " + str2);
// Display the common ending between the two strings
Console.WriteLine("\nCommon ending between said two strings:" + test(str1, str2));
// Change the values of str1 and str2
str1 = "thisisatest";
str2 = "testing123testing";
// Display the new original strings
Console.WriteLine("\nOriginal strings: " + str1 + " " + str2);
// Display the common ending between the two strings
Console.WriteLine("\nCommon ending between said two strings:" + test(str1, str2));
}
// Define a method 'test' that takes two string arguments and returns the common ending between them
public static string test(string st1, string st2)
{
// Loop through the characters of st1
for (int i = 0; i < st1.Length; i++)
{
// Get the substring of st1 starting from index i
string end = st1.Substring(i);
// Check if st2 ends with the obtained substring of st1
if (st2.EndsWith(end))
{
// Return the common ending
return end;
}
}
// If no common ending is found, return an empty string
return "";
}
}
}
Sample Output:
Original strings: running ruminating Common ending between said two strings:ing Original strings: thisisatest testing123testing Common ending between said two strings:
Flowchart :
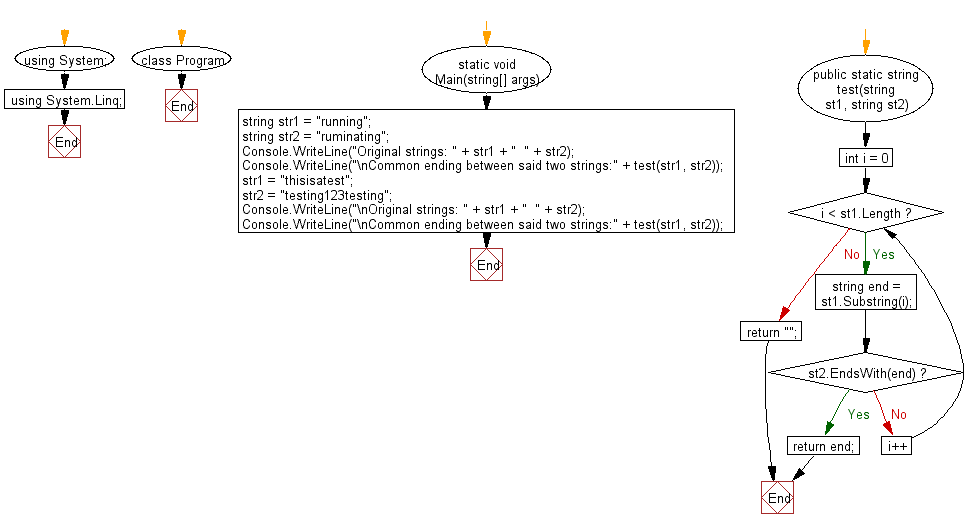
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous : Reverse all the words of a given string which have even length.
Next: Reverse the words of three or more lengths in a string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/csharp-exercises/string/csharp-string-exercise-56.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics