C# Sharp Exercises: Reverse the words of three or more lengths in a string
Write a C# Sharp program that takes a string and reverses the words of three or more lengths in a string. Return the updated string. As input characters, only letters and spaces are permitted.
Sample Data:
("The quick brown fox jumps over the lazy dog") -> "ehT kciuq nworb xof spmuj revo eht yzal god"
("Reverse the words of three or more") -> "esreveR eht sdrow of eerht or erom"
("ABcDef") -> "feDcBA"
Sample Solution:
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
// Declare and initialize a string variable
string text = "The quick brown fox jumps over the lazy dog";
// Display the original string
Console.WriteLine("Original strings: " + text);
// Display the action about to be performed
Console.WriteLine("\nReverse the words of three or more lengths of the said string:");
// Invoke the 'test' method and display the modified string
Console.WriteLine(test(text));
}
// Define a method 'test' that takes a string argument 'text' and returns a modified string
public static string test(string text)
{
// Split the input text into words, check if the word length is three or more
// If the length is three or more, reverse the characters of the word; otherwise, keep the word as it is
return string.Join(" ", text.Split(' ').Select(x => x.Length >= 3 ? new string(x.Reverse().ToArray()) : new string(x.ToArray())));
}
}
}
Sample Output:
Original strings: The quick brown fox jumps over the lazy dog Reverse the words of three or more lengths of the said string: ehT kciuq nworb xof spmuj revo eht yzal god
Flowchart :
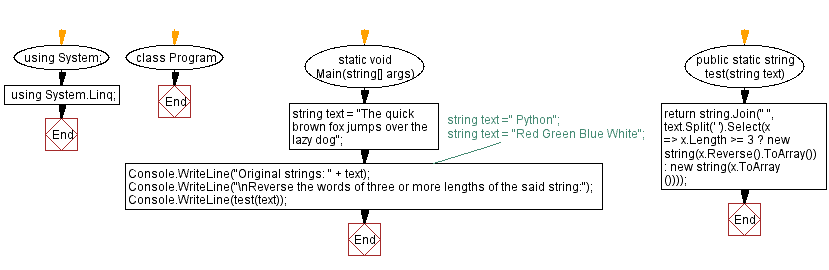
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Find the longest common ending between two strings.
Next: Check if a string is an anagram of another given string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.