C#: Longest abecedarian word in a array of words
Abecedarian Word:
The English noun and adjective abecedarian has several closely related senses. As a noun, it means "someone learning the letters of the alphabet," and more loosely, "a beginner in a field of learning." As an adjective, abecedarian means "pertaining to the alphabet; arranged in alphabetical order; elementary, rudimentary."
Write a C# Sharp program to find the longest abecedarian word in a given array of words.
Sample Data:
({"abc", "abcd", "abcdef", "pqrstuv"}) -> "pqrstuv"
({"abc", "abcd", "abcdef", "pqrs"}) -> "abcdef"
({}) -> "Empty array!"
Sample Solution:
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
// Declare and initialize an array of words
string[] words = {"abc", "abcd", "abcdef", "pqrstuv"};
// Display the original array of words
Console.WriteLine("Original array of words: ");
Console.WriteLine($"{string.Join(", ", words)}");
// Display the longest abecedarian word in the array
Console.WriteLine("Longest abecedarian word in the said array of words: " + test(words));
// Change the array of words
string[] words1 = {"abc", "abcd", "abcdef", "pqrs"};
// Display the new original array of words
Console.WriteLine("\nOriginal array of words: ");
Console.WriteLine($"{string.Join(", ", words1)}");
// Display the longest abecedarian word in the array
Console.WriteLine("Longest abecedarian word in the said array of words: " + test(words1));
// Change the array of words to an empty array
string[] words2 = {};
// Display the new original array of words (empty)
Console.WriteLine("\nOriginal array of words: ");
Console.WriteLine($"{string.Join(", ", words2)}");
// Display a message for an empty array or the longest abecedarian word in the array
Console.WriteLine("Longest abecedarian word in the said array of words: " + test(words2));
}
// Define a method 'test' that takes an array of strings 'array_words' and returns a string
public static string test(string[] array_words)
{
// Check if the array is empty
if (!array_words.Any())
{
return "Empty array!";
}
// Initialize an empty string to store the result
string result = "";
// Iterate through each word in the array
foreach (string word in array_words)
{
// Convert the word to a character array and sort it
char[] temp = word.ToCharArray();
Array.Sort(temp);
// Create a string from the sorted characters
string cword = new string(temp);
// Check if the sorted word is equal to the original word and its length is greater than the current result's length
if (cword == word && word.Length > result.Length)
result = word;
}
// Return the longest abecedarian word found in the array
return result;
}
}
}
Sample Output:
Original array of words: abc, abcd, abcdef, pqrstuv Longest abecedarian word in the said array of words: pqrstuv Original array of words: abc, abcd, abcdef, pqrs Longest abecedarian word in the said array of words: abcdef Original array of words: Longest abecedarian word in the said array of words: Empty array!
Flowchart :
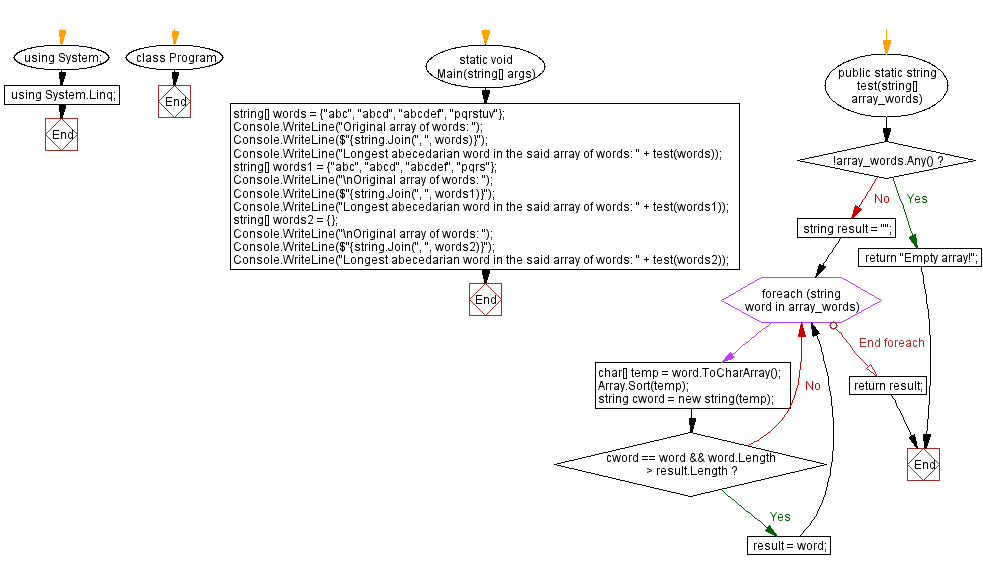
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous C# Sharp Exercise: Number of times a substring appeared in a string.
Next C# Sharp Exercise: Find the century from a given year.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.