C#: Find the century from a given year
From Wikipedia –
A century is a period of 100 years. Centuries are numbered ordinally in English and many other languages. The word century comes from the Latin centum, meaning one hundred. Century is sometimes abbreviated as c.
Although a century can mean any arbitrary period of 100 years, there are two viewpoints on the nature of standard centuries. One is based on strict construction, while the other is based on popular perception.
According to the strict construction, the 1st century AD began with AD 1 and ended with AD 100, the 2nd century spanning the years 101 to 200, with the same pattern continuing onward. In this model, the n-th century starts with the year that ends with "01", and ends with the year that ends with "00"; for example, the 20th century comprises the years 1901 to 2000 in strict usage.
In popular perception and practice, centuries are structured by grouping years based on sharing the 'hundreds' digit(s). In this model, the n-th century starts with the year that ends in "00" and ends with the year ending in "99"; for example, the years 1900 to 1999, in popular culture, constitute the 20th century.
Write a C# Sharp program to find the century of a given year.
Sample Data:
(1435) -> "15th century"
(1567) -> "16th century"
(1921) -> "20th century"
(2014) -> "21st century"
Sample Solution:
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
// Declare and initialize an integer variable 'n'
int n = 1435;
// Display the original year
Console.WriteLine("Original year: " + n);
// Display the century of the said year
Console.WriteLine("Century of the said year: " + test(n));
// Change the value of 'n'
n = 1567;
// Display the new original year
Console.WriteLine("\nOriginal year: " + n);
// Display the century of the said year
Console.WriteLine("Century of the said year: " + test(n));
// Change the value of 'n'
n = 1921;
// Display the new original year
Console.WriteLine("\nOriginal year: " + n);
// Display the century of the said year
Console.WriteLine("Century of the said year: " + test(n));
// Change the value of 'n'
n = 2014;
// Display the new original year
Console.WriteLine("\nOriginal year: " + n);
// Display the century of the said year
Console.WriteLine("Century of the said year: " + test(n));
}
// Define a method 'test' that takes an integer argument 'year' and returns a string
public static string test(int year)
{
// Calculate the century of the given year based on the modulo operation
int n = year % 100 == 0 ? year / 100 : year / 100 + 1;
// Check if the century is greater than 20
if (n > 20)
return n + "st century"; // Return the century with the appropriate suffix
else
return n + "th century"; // Return the century with the appropriate suffix
}
}
}
Sample Output:
Original year: 1435 Century of the said year: 15th century Original year: 1567 Century of the said year: 16th century Original year: 1921 Century of the said year: 20th century Original year: 2014 Century of the said year: 21st century
Flowchart :
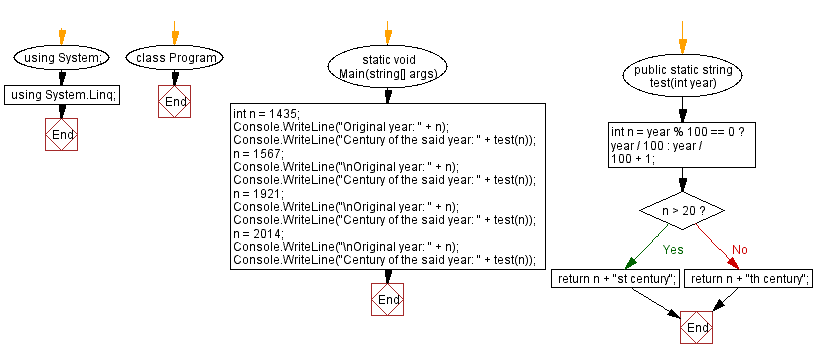
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous C# Sharp Exercise: Longest abecedarian word in a array of words.
Next C# Sharp Exercise: Highest frequency character(s) in the words of a string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.