C#: Highest frequency character(s) in the words of a string
Write a C# Sharp program to calculate the highest frequency of the character(s) in the words of a given string.
Sample Data:
("aaaaaa") -> "a"
("Century of the said year") -> ""
("CPP Exercises”) -> "s, e, p"
Sample Solution:
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
// Declare and initialize a string variable 'text'
string text = "aaaaaa";
// Display the original string
Console.WriteLine("Original strings: " + text);
// Display the highest frequency character(s) in the string
Console.WriteLine("Highest Frequency Character(s) in the said String: " + test(text));
// Change the value of 'text'
text = "Century of the said year";
// Display the new original string
Console.WriteLine("\nOriginal strings: " + text);
// Display the highest frequency character(s) in the string
Console.WriteLine("Highest Frequency Character(s) in the said String: " + test(text));
// Change the value of 'text'
text = "CPP Exercises";
// Display the new original string
Console.WriteLine("\nOriginal strings: " + text);
// Display the highest frequency character(s) in the string
Console.WriteLine("Highest Frequency Character(s) in the said String: " + test(text));
}
// Define a method 'test' that takes a string argument 'text' and returns a string
public static string test(string text)
{
// Group the characters in reverse order by their frequency, ordered by count in descending order
var groups = text.Reverse().GroupBy(ch => ch).OrderByDescending(el => el.Count());
// Get the maximum occurrence of characters
int max_occurs = groups.First().Count();
// If the maximum occurrence is 1, return a message indicating all unique characters
if (max_occurs == 1)
return "All unique characters";
// Otherwise, return the characters with the highest frequency
return string.Join(", ", groups.TakeWhile(el => el.Count() == max_occurs).Select(el => el.Key));
}
}
}
Sample Output:
Original strings: aaaaaa Highest Frequency Character(s) in the said String: a Original strings: Century of the said year Highest Frequency Character(s) in the said String: Original strings: CPP Exercises Highest Frequency Character(s) in the said String: s, e, P
Flowchart :
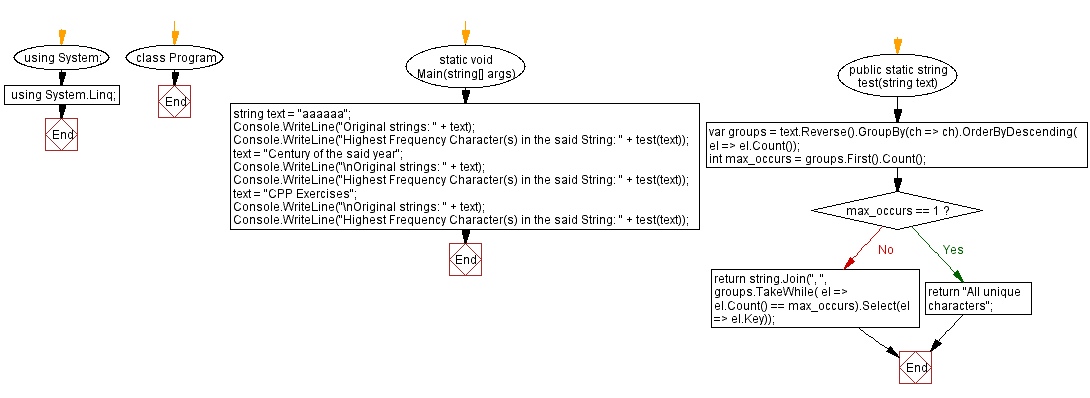
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous C# Sharp Exercise: Find the century from a given year.
Next C# Sharp Exercise: Convert ASCII text to hexadecimal value as a string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.