C#: Convert ASCII text to hexadecimal value as a string
Write a C# Sharp program that converts ASCII characters to hexadecimal values.
Sample Data:
("Python") -> "50 79 74 68 6f 6e"
("Century of the year") -> 43 65 6e 74 75 72 79 20 6f 66 20 74 68 65 20 79 65 61 72
("CPP Exercises") -> 43 50 50 20 45 78 65 72 63 69 73 65 73
Sample Solution:
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
// Declare and initialize a string variable 'text'
string text = "Python";
// Display the original string
Console.WriteLine("Original strings: " + text);
// Display the conversion of ASCII text to hexadecimal value as a string
Console.WriteLine("Convert said ASCII text to hexadecimal value as a string:" + test(text));
// Change the value of 'text'
text = "Century of the year";
// Display the new original string
Console.WriteLine("\nOriginal strings: " + text);
// Display the conversion of ASCII text to hexadecimal value as a string
Console.WriteLine("Convert said ASCII text to hexadecimal value as a string:" + test(text));
// Change the value of 'text'
text = "CPP Exercises";
// Display the new original string
Console.WriteLine("\nOriginal strings: " + text);
// Display the conversion of ASCII text to hexadecimal value as a string
Console.WriteLine("Convert said ASCII text to hexadecimal value as a string:" + test(text));
}
// Define a method 'test' that takes a string argument 'text' and returns a string
public static string test(string text)
{
// Convert each character in the string to its ASCII code, then to its hexadecimal representation
// Aggregate the hexadecimal representations of each character into a single string
// Separate each hexadecimal value with a space, convert to lowercase, and trim any leading/trailing spaces
return text.ToCharArray().Aggregate("", (a, b) => a + ((byte)b).ToString("X") + " ").ToLower().Trim();
}
}
}
Sample Output:
Original strings: Python Convert said ASCII text to hexadecimal value as a string:50 79 74 68 6f 6e Original strings: Century of the year Convert said ASCII text to hexadecimal value as a string:43 65 6e 74 75 72 79 20 6f 66 20 74 68 65 20 79 65 61 72 Original strings: CPP Exercises Convert said ASCII text to hexadecimal value as a string:43 50 50 20 45 78 65 72 63 69 73 65 73
Flowchart :
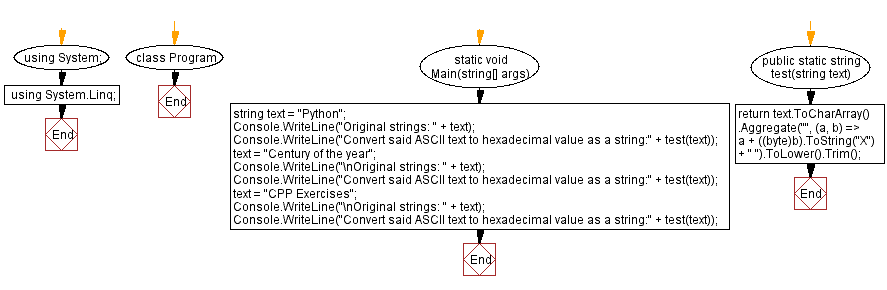
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous C# Sharp Exercise: Highest frequency character(s) in the words of a string.
Next C# Sharp Exercise: Check if a string has all unique characters.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.